The Python zip function is a built-in function that takes two or more iterables as arguments and returns an iterator that aggregates the elements from each of the iterables. The resulting iterator contains tuples where the i-th tuple contains the i-th element from each of the input iterables. If the input iterables are of different lengths, the resulting iterator will have a length equal to the shortest input iterable. The zip function is commonly used to combine two or more lists or tuples into a single iterable for processing. Keep reading below to learn how to python zip in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
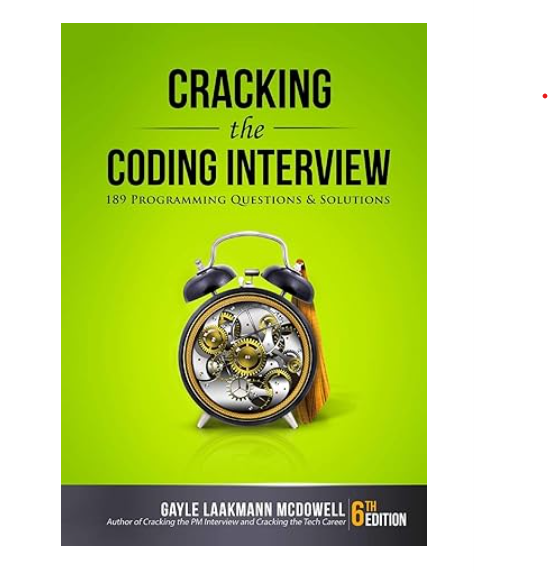
Python ‘zip’ in Rust With Example Code
Python’s `zip` function is a powerful tool for working with multiple iterables at once. Rust, being a systems programming language, also has a similar functionality for working with iterators called `zip`. In this blog post, we will explore how to use `zip` in Rust to achieve similar functionality as Python’s `zip`.
To use `zip` in Rust, we first need to import the `Iterator` trait. This trait provides the `zip` method that we can use to combine two or more iterators into a single iterator. Here’s an example:
let numbers = vec![1, 2, 3];
let letters = vec!['a', 'b', 'c'];
for (number, letter) in numbers.iter().zip(letters.iter()) {
println!("{}{}", number, letter);
}
In this example, we have two vectors `numbers` and `letters`. We use the `iter` method to get an iterator for each vector and then use the `zip` method to combine them into a single iterator. We then use a `for` loop to iterate over the combined iterator and print out each pair of numbers and letters.
One thing to note is that the `zip` method returns an iterator that stops when the shortest input iterator is exhausted. This means that if one of the input iterators is shorter than the others, the combined iterator will stop when that iterator is exhausted.
In conclusion, Rust’s `zip` method provides similar functionality as Python’s `zip` function for working with multiple iterators at once. By using the `Iterator` trait and the `zip` method, we can easily combine two or more iterators into a single iterator and work with them in a concise and efficient manner.
Equivalent of Python zip in Rust
In conclusion, the Rust programming language provides a powerful and efficient alternative to Python’s zip function with its own zip implementation. The zip function in Rust allows developers to combine multiple iterators into a single iterator, making it easier to work with complex data structures. Additionally, Rust’s zip function is designed to be memory-efficient, making it ideal for handling large datasets. With its strong focus on performance and safety, Rust is quickly becoming a popular choice for developers who want to build high-performance applications. So, if you’re looking for a fast and reliable way to zip iterators in Rust, the zip function is definitely worth exploring.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |