An array is a fundamental data structure in computer science that stores a collection of elements of the same data type in contiguous memory locations. It is a fixed-size data structure that allows for efficient access to individual elements using an index. Arrays are commonly used for storing and manipulating data in algorithms and programs, such as sorting and searching algorithms. They are also used in many programming languages as a basic building block for more complex data structures, such as lists, stacks, and queues. However, arrays have limitations, such as fixed size and the need for contiguous memory, which can make them less flexible in certain situations. Keep reading below to learn how to use a Array in Bash.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
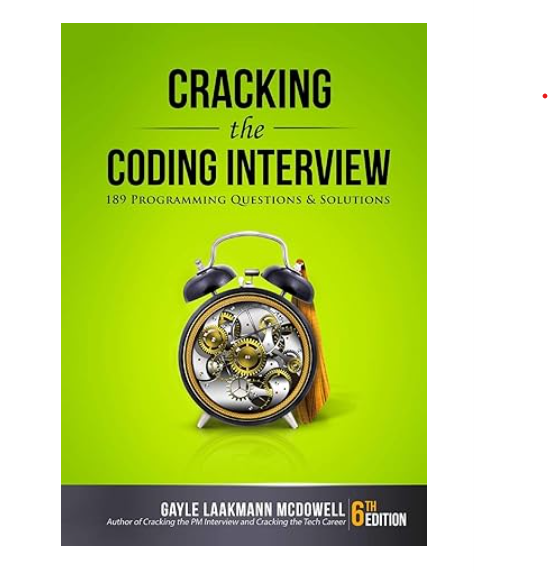
How to use a Array in Bash with example code
Arrays are a useful feature in Bash that allow you to store multiple values in a single variable. In this blog post, we will explore how to use arrays in Bash with example code.
To declare an array in Bash, you can use the following syntax:
my_array=(value1 value2 value3)
This creates an array called my_array
with three values: value1
, value2
, and value3
.
You can access individual elements of the array using their index. The first element has an index of 0, the second has an index of 1, and so on. To access the first element of my_array
, you can use the following syntax:
echo ${my_array[0]}
This will output value1
.
You can also loop through all the elements of an array using a for
loop. Here’s an example:
my_array=(apple banana cherry)
for fruit in ${my_array[@]}
do
echo $fruit
done
This will output:
apple
banana
cherry
You can also add elements to an array using the +=
operator. Here’s an example:
my_array=(apple banana cherry)
my_array+=(date)
echo ${my_array[@]}
This will output:
apple banana cherry date
In conclusion, arrays are a powerful feature in Bash that allow you to store multiple values in a single variable. With the examples provided in this blog post, you should be able to start using arrays in your Bash scripts.
What is a Array in Bash?
In conclusion, an array in Bash is a collection of elements that are stored under a single variable name. It allows for easy manipulation and organization of data, making it a powerful tool for scripting and automation tasks. Arrays can be created and accessed using various methods, including the use of indexes and loops. With the ability to store multiple values in a single variable, arrays provide a convenient way to manage and process data in Bash scripts. Whether you are a beginner or an experienced Bash user, understanding arrays is an essential skill that can greatly enhance your scripting capabilities.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |