An array is a fundamental data structure in computer science that stores a collection of elements of the same data type in contiguous memory locations. It is a fixed-size data structure that allows for efficient access to individual elements using an index. Arrays are commonly used for storing and manipulating data in algorithms and programs, such as sorting and searching algorithms. They are also used in many programming languages as a basic building block for more complex data structures, such as lists, stacks, and queues. However, arrays have limitations, such as fixed size and the need for contiguous memory, which can make them less flexible than other data structures in certain situations. Keep reading below to learn how to use a Array in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
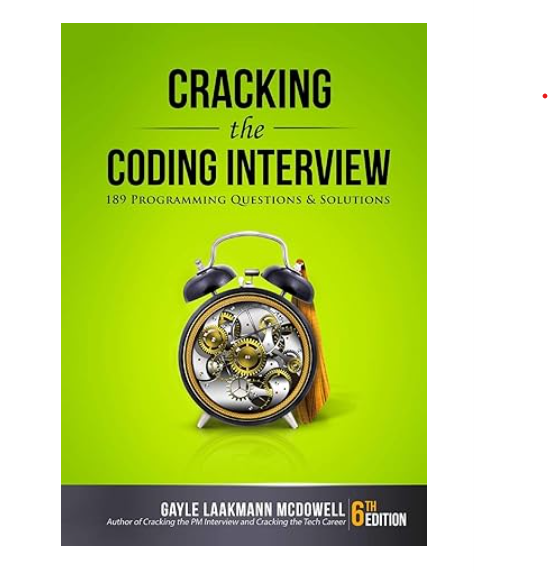
How to use a Array in C# with example code
Arrays are an essential part of programming in C#. They allow you to store multiple values of the same data type in a single variable. In this blog post, we will discuss how to use arrays in C# and provide some example code to help you get started.
Declaring an Array
To declare an array in C#, you need to specify the data type of the elements in the array and the number of elements in the array. Here is an example of how to declare an array of integers with five elements:
int[] numbers = new int[5];
In this example, we are declaring an array of integers called “numbers” with five elements. The “new” keyword is used to create a new instance of the array with the specified number of elements.
Initializing an Array
Once you have declared an array, you can initialize it with values. Here is an example of how to initialize the “numbers” array with values:
int[] numbers = new int[] { 1, 2, 3, 4, 5 };
In this example, we are initializing the “numbers” array with the values 1, 2, 3, 4, and 5. The curly braces are used to enclose the values.
Accessing Array Elements
You can access individual elements in an array by using the index of the element. The index of the first element in an array is 0. Here is an example of how to access the third element in the “numbers” array:
int thirdNumber = numbers[2];
In this example, we are accessing the third element in the “numbers” array by using the index 2. The value of the third element (which is 3) is assigned to the variable “thirdNumber”.
Looping Through an Array
You can use a loop to iterate through all the elements in an array. Here is an example of how to loop through the “numbers” array and print out each element:
for (int i = 0; i < numbers.Length; i++)
{
Console.WriteLine(numbers[i]);
}
In this example, we are using a "for" loop to iterate through all the elements in the "numbers" array. The "Length" property is used to get the number of elements in the array. The value of each element is printed out to the console.
Conclusion
Arrays are a powerful tool in C# programming. They allow you to store multiple values of the same data type in a single variable. By declaring, initializing, accessing, and looping through arrays, you can create more efficient and effective code.
What is a Array in C#?
In conclusion, an array in C# is a powerful data structure that allows programmers to store and manipulate a collection of elements of the same data type. It provides a convenient way to access and modify data in a sequential manner, making it an essential tool for many programming tasks. With its flexibility and efficiency, arrays are widely used in various applications, from simple data processing to complex algorithms. Understanding the basics of arrays in C# is crucial for any programmer who wants to develop efficient and effective software. By mastering this fundamental concept, you can unlock the full potential of C# and create robust and scalable applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |