An array is a fundamental data structure in computer science that stores a collection of elements of the same data type in contiguous memory locations. It is a fixed-size data structure that allows for efficient access to individual elements using an index. Arrays can be one-dimensional, two-dimensional, or multi-dimensional, and can be initialized with a set of values or left empty. They are commonly used in algorithms and programs for storing and manipulating data, such as sorting and searching algorithms, and are an essential concept in computer science. Keep reading below to learn how to use a Array in PHP.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
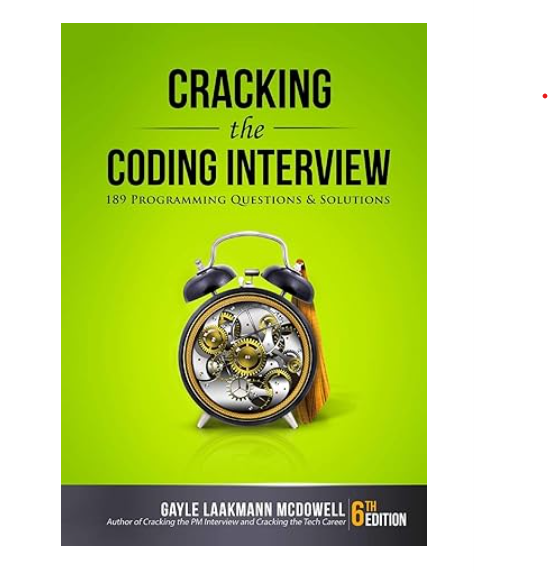
How to use a Array in PHP with example code
Arrays are an essential data structure in PHP. They allow you to store multiple values in a single variable, making it easier to manage and manipulate data. In this blog post, we will explore how to use arrays in PHP and provide some example code to help you get started.
Creating an Array
To create an array in PHP, you can use the array() function. This function takes any number of comma-separated values and returns an array containing those values. For example, the following code creates an array with three values:
$myArray = array("apple", "banana", "orange");
Accessing Array Elements
You can access individual elements of an array using their index. In PHP, array indexes start at 0. For example, to access the first element of the $myArray array, you would use the following code:
$firstElement = $myArray[0];
You can also change the value of an array element by assigning a new value to its index. For example, the following code changes the value of the second element of the $myArray array to “grape”:
$myArray[1] = "grape";
Looping Through an Array
To loop through all the elements of an array, you can use a for loop. The loop should start at 0 and end at the length of the array minus one. For example, the following code loops through all the elements of the $myArray array and prints them to the screen:
for ($i = 0; $i < count($myArray); $i++) {
echo $myArray[$i] . "
";
}
Multidimensional Arrays
PHP also supports multidimensional arrays, which are arrays of arrays. You can create a multidimensional array by nesting arrays inside other arrays. For example, the following code creates a two-dimensional array:
$myArray = array(
array("apple", "banana", "orange"),
array("carrot", "pepper", "broccoli")
);
You can access elements of a multidimensional array using multiple indexes. For example, the following code accesses the element in the first row and second column of the $myArray array:
$element = $myArray[0][1];
Conclusion
Arrays are a powerful tool in PHP that allow you to store and manipulate multiple values in a single variable. By understanding how to create, access, and loop through arrays, you can write more efficient and effective PHP code.
What is a Array in PHP?
In conclusion, an array in PHP is a powerful data structure that allows developers to store and manipulate multiple values in a single variable. It is a fundamental concept in PHP programming and is used extensively in various applications. With arrays, developers can easily access and modify data, making it an essential tool for building dynamic and interactive web applications. Whether you are a beginner or an experienced PHP developer, understanding arrays is crucial to writing efficient and effective code. So, if you are looking to improve your PHP skills, mastering arrays should be at the top of your list.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |