An array is a fundamental data structure in computer science that stores a collection of elements of the same data type in contiguous memory locations. It is a fixed-size data structure that allows for efficient access to individual elements using an index. Arrays can be one-dimensional, two-dimensional, or multi-dimensional, and can be initialized with a set of values or left empty. They are commonly used in algorithms and programs for storing and manipulating data, such as sorting and searching algorithms, and are an essential concept in computer science. Keep reading below to learn how to use a Array in Python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
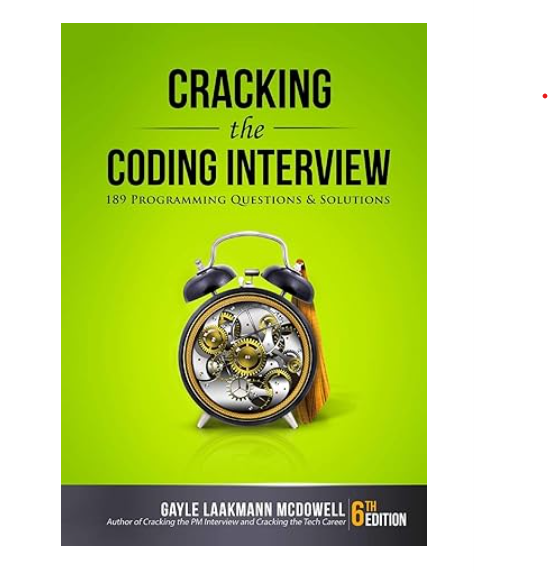
How to use a Array in Python with example code
Arrays are a fundamental data structure in Python that allow you to store and manipulate collections of data. In this blog post, we will explore how to use arrays in Python and provide some example code to help you get started.
To use arrays in Python, you first need to import the `array` module. This module provides a way to create arrays of a specific data type, such as integers or floating-point numbers. Here is an example of how to create an array of integers:
import array
my_array = array.array('i', [1, 2, 3, 4, 5])
In this example, we create an array of integers using the `array` function and passing in the data type `’i’` and a list of integers `[1, 2, 3, 4, 5]`. The data type `’i’` specifies that we want an array of integers.
Once you have created an array, you can access its elements using indexing. Array indexing in Python starts at 0, so the first element of an array is at index 0. Here is an example of how to access the first element of the array we created earlier:
print(my_array[0])
This will output `1`, which is the first element of the array.
You can also modify the elements of an array by assigning new values to them. Here is an example of how to change the second element of the array we created earlier to a new value:
my_array[1] = 10
print(my_array)
This will output `[1, 10, 3, 4, 5]`, which is the modified array with the second element changed to `10`.
In addition to creating and modifying arrays, you can also perform various operations on them, such as sorting or searching. The `array` module provides several functions for these operations, such as `sort` and `index`. Here is an example of how to sort the array we created earlier:
my_array.sort()
print(my_array)
This will output `[1, 3, 4, 5, 10]`, which is the sorted array.
In conclusion, arrays are a powerful data structure in Python that allow you to store and manipulate collections of data. By importing the `array` module and using its functions, you can create, modify, and perform operations on arrays with ease.
What is a Array in Python?
In conclusion, an array in Python is a data structure that allows you to store a collection of elements of the same data type. It is a powerful tool that can be used to efficiently manage and manipulate large amounts of data. Arrays in Python are versatile and can be used in a variety of applications, from scientific computing to web development. Understanding how to use arrays in Python is an essential skill for any programmer looking to work with data. With its simplicity and flexibility, Python’s array module is a great choice for anyone looking to work with arrays in their code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |