An array is a fundamental data structure in computer science that stores a collection of elements of the same data type in contiguous memory locations. It is a fixed-size data structure that allows for efficient access to individual elements using an index. Arrays are commonly used in algorithms and programs to store and manipulate data, such as lists of numbers, characters, or objects. They are also used to implement other data structures, such as stacks, queues, and matrices. However, arrays have limitations, such as fixed size and the need to shift elements when inserting or deleting, which have led to the development of more advanced data structures. Keep reading below to learn how to use a Array in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
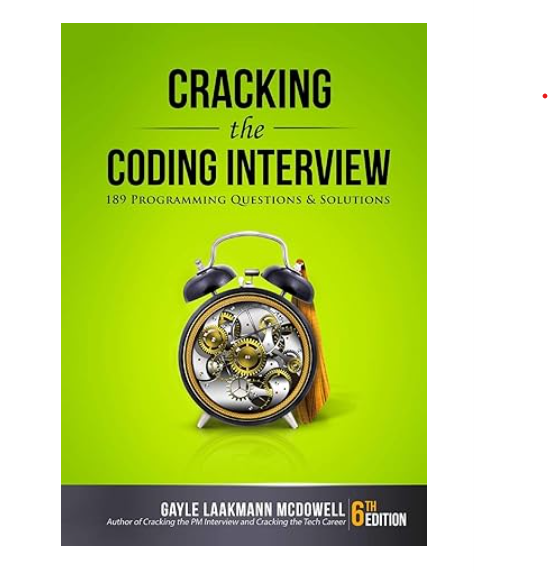
How to use a Array in Rust with example code
Arrays are a fundamental data structure in Rust that allow you to store a fixed-size sequence of elements of the same type. In this blog post, we will explore how to use arrays in Rust with example code.
Declaring an array in Rust is straightforward. You can declare an array by specifying the type of the elements it will contain, followed by the number of elements in the array, enclosed in square brackets. For example, to declare an array of 5 integers, you would write:
let my_array: [i32; 5] = [1, 2, 3, 4, 5];
In this example, we declare an array called `my_array` that contains 5 integers. The type of the array is `[i32; 5]`, which means it contains 5 elements of type `i32`.
You can also initialize an array with a default value for all its elements. To do this, you can use the syntax `[default_value; size]`. For example, to declare an array of 3 booleans initialized to `false`, you would write:
let my_array: [bool; 3] = [false; 3];
In this example, we declare an array called `my_array` that contains 3 booleans initialized to `false`.
Accessing elements in an array is done using square brackets and the index of the element you want to access. The index of the first element in an array is 0. For example, to access the first element of `my_array`, you would write:
let first_element = my_array[0];
In this example, we assign the value of the first element of `my_array` to a variable called `first_element`.
Arrays in Rust are fixed-size, which means you cannot add or remove elements from an array once it has been declared. However, you can modify the value of an element in an array by assigning a new value to it. For example, to change the value of the second element of `my_array` to `true`, you would write:
my_array[1] = true;
In this example, we change the value of the second element of `my_array` to `true`.
In conclusion, arrays are a powerful data structure in Rust that allow you to store a fixed-size sequence of elements of the same type. They are easy to declare and initialize, and you can access and modify their elements using simple syntax.
What is a Array in Rust?
In conclusion, an array in Rust is a fixed-size collection of elements of the same data type. It is a fundamental data structure that is widely used in programming to store and manipulate data efficiently. Rust provides a safe and efficient way to work with arrays, with features such as bounds checking and memory safety. With Rust’s powerful array manipulation capabilities, developers can easily create, modify, and access arrays to build robust and efficient applications. Whether you are a beginner or an experienced programmer, understanding arrays in Rust is essential to mastering the language and building high-quality software.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |