An array is a fundamental data structure in computer science that stores a collection of elements of the same data type in contiguous memory locations. It is a fixed-size data structure that allows for efficient access to individual elements using an index. Arrays can be one-dimensional, two-dimensional, or multi-dimensional, and can be initialized with a set of values or left empty. They are commonly used in algorithms and programs for storing and manipulating data, such as sorting and searching algorithms, and are an essential concept in computer science. Keep reading below to learn how to use a Array in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
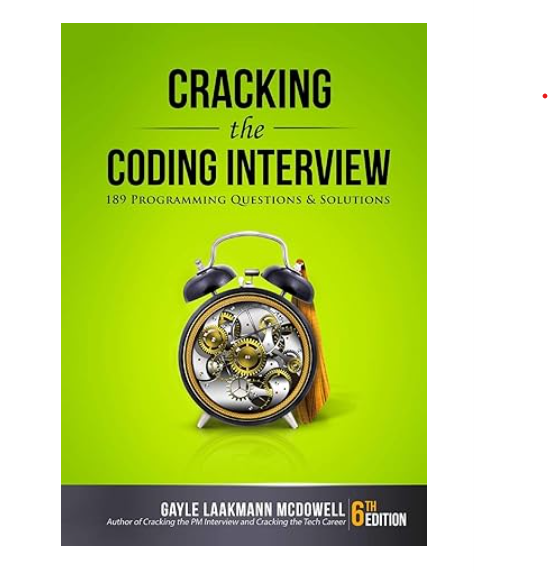
How to use a Array in TypeScript with example code
Arrays are an essential part of any programming language, and TypeScript is no exception. In TypeScript, arrays are a collection of elements of the same type. They are used to store and manipulate data efficiently. In this blog post, we will discuss how to use arrays in TypeScript with example code.
Declaring an array in TypeScript is similar to declaring a variable. To declare an array, we use the square brackets notation. For example, to declare an array of numbers, we can use the following code:
let numbers: number[];
This code declares an array of numbers named “numbers”. We can also initialize the array with values. For example:
let numbers: number[] = [1, 2, 3, 4, 5];
This code initializes the “numbers” array with the values 1, 2, 3, 4, and 5.
To access the elements of an array, we use the index notation. The index of the first element in an array is 0. For example, to access the first element of the “numbers” array, we can use the following code:
let firstNumber = numbers[0];
This code assigns the value of the first element of the “numbers” array to the variable “firstNumber”.
We can also use loops to iterate over the elements of an array. For example, to print all the elements of the “numbers” array, we can use the following code:
for (let i = 0; i < numbers.length; i++) {
console.log(numbers[i]);
}
This code iterates over the "numbers" array using a for loop and prints each element to the console.
In addition to the basic operations, TypeScript provides many built-in methods to manipulate arrays. For example, we can use the "push" method to add an element to the end of an array. For example:
numbers.push(6);
This code adds the value 6 to the end of the "numbers" array.
In conclusion, arrays are an essential part of TypeScript programming. They are used to store and manipulate data efficiently. In this blog post, we discussed how to declare and initialize arrays, access their elements, iterate over them using loops, and use built-in methods to manipulate them.
What is a Array in TypeScript?
In conclusion, an array in TypeScript is a powerful data structure that allows developers to store and manipulate collections of data in a simple and efficient manner. With its ability to hold multiple values of the same type, arrays are an essential tool for building complex applications that require the management of large amounts of data. TypeScript provides developers with a range of features and syntax for working with arrays, including methods for adding, removing, and manipulating elements, as well as powerful iteration and filtering capabilities. By understanding the basics of arrays in TypeScript, developers can unlock the full potential of this versatile data structure and build more robust and efficient applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |