A cache is a data structure used in computer science to store frequently accessed data in a faster and more efficient way. It is typically used to improve the performance of a system by reducing the time it takes to access data that is frequently used. The cache works by storing a copy of the data in a faster and more accessible location, such as in memory, so that it can be retrieved quickly when needed. When data is requested, the cache is checked first, and if the data is found, it is returned immediately. If the data is not found in the cache, it is retrieved from the slower storage location and added to the cache for future use. Keep reading below to learn how to use a Cache in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
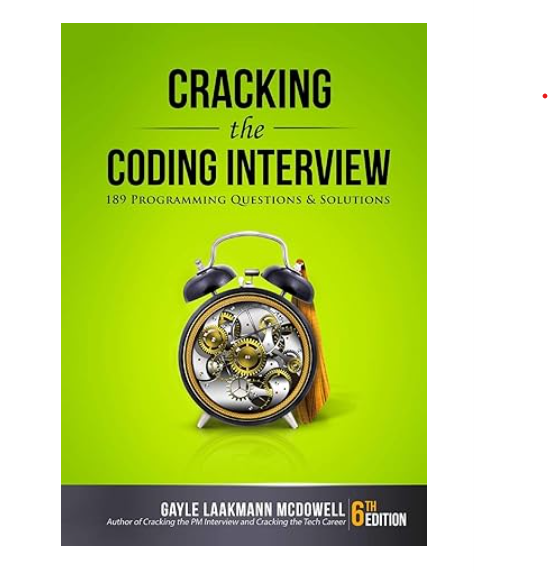
How to use a Cache in C++ with example code
Caching is a technique used to improve the performance of an application by storing frequently accessed data in a temporary storage area. In C++, caching can be implemented using various data structures such as arrays, maps, and hash tables. In this blog post, we will discuss how to use a cache in C++ with an example code.
To implement a cache in C++, we can use a map data structure from the STL library. The map data structure stores key-value pairs and provides fast access to the values based on the keys. We can use this property of the map to store frequently accessed data in the cache.
Let’s consider an example where we have a function that calculates the factorial of a number. The factorial of a number is the product of all the numbers from 1 to that number. For example, the factorial of 5 is 5*4*3*2*1 = 120. The function to calculate the factorial of a number can be implemented using recursion as follows:
int factorial(int n) {
if (n == 0) {
return 1;
}
return n * factorial(n - 1);
}
Now, let’s assume that we need to calculate the factorial of the same number multiple times in our application. Instead of recalculating the factorial every time, we can store the result in a cache and retrieve it when needed. We can implement the cache using a map as follows:
std::map
int factorial(int n) {
if (n == 0) {
return 1;
}
if (cache.find(n) != cache.end()) {
return cache[n];
}
int result = n * factorial(n - 1);
cache[n] = result;
return result;
}
In the above code, we first check if the result for the given number is already present in the cache. If it is present, we return the cached result. If not, we calculate the result using recursion and store it in the cache for future use.
Using a cache can significantly improve the performance of our application by reducing the number of calculations needed. However, we need to be careful while using a cache as it can consume a lot of memory if not used properly. We should only store frequently accessed data in the cache and limit the size of the cache to avoid consuming too much memory.
In conclusion, caching is a powerful technique that can be used to improve the performance of our C++ applications. We can implement a cache using various data structures, and the map data structure from the STL library is a good choice for implementing a cache. By using a cache, we can reduce the number of calculations needed and improve the overall performance of our application.
What is a Cache in C++?
In conclusion, a cache in C++ is a temporary storage area that stores frequently accessed data to improve the performance of a program. It is a crucial component of modern computer systems that helps reduce the time it takes to access data from the main memory. C++ provides several cache optimization techniques, such as cache blocking and prefetching, to help programmers optimize their code for better cache performance. Understanding how the cache works and how to optimize code for it can significantly improve the performance of C++ programs. By utilizing the cache effectively, programmers can create faster and more efficient programs that can handle large amounts of data with ease.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |