A cache is a data structure used in computer science to store frequently accessed data in a faster and more efficient way. It is typically used to improve the performance of a system by reducing the time it takes to access data that is frequently used. The cache works by storing a copy of the data in a faster and more accessible location, such as in memory, so that it can be retrieved quickly when needed. When data is requested, the cache is checked first, and if the data is found, it is returned immediately. If the data is not found in the cache, it is retrieved from the slower storage location and added to the cache for future use. Keep reading below to learn how to use a Cache in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
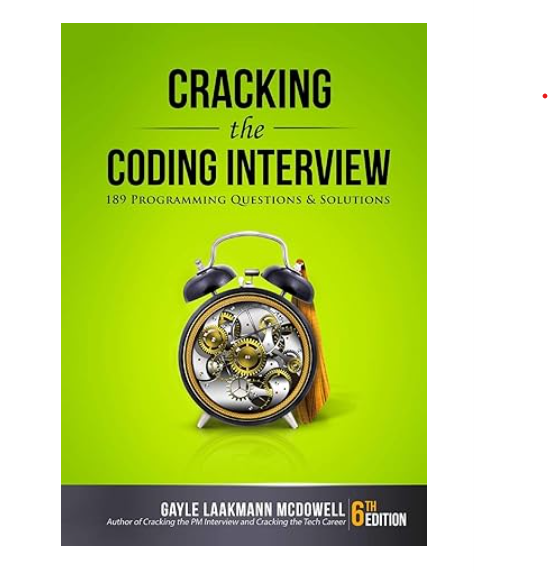
How to use a Cache in Go with example code
Caching is an essential technique for improving the performance of web applications. In Go, caching can be implemented using various libraries and techniques. In this blog post, we will explore how to use a cache in Go with an example code.
One of the popular caching libraries in Go is “go-cache”. It is a simple, thread-safe, in-memory caching library that can be used to cache any type of data. To use this library, we need to install it using the following command:
go get github.com/patrickmn/go-cache
Once the library is installed, we can start using it in our code. Here is an example code that demonstrates how to use the “go-cache” library:
import (
"github.com/patrickmn/go-cache"
"time"
)
func main() {
// Create a new cache with a default expiration time of 5 minutes, and which
// purges expired items every 10 minutes
c := cache.New(5*time.Minute, 10*time.Minute)
// Set the value of the key "foo" to "bar", with an expiration time of 1 minute
c.Set("foo", "bar", cache.DefaultExpiration)
// Get the value of the key "foo" from the cache
if x, found := c.Get("foo"); found {
fmt.Println(x)
}
// Delete the key "foo" from the cache
c.Delete("foo")
}
In the above code, we first import the “go-cache” library and the “time” package. We then create a new cache with a default expiration time of 5 minutes and a purge time of 10 minutes. We set the value of the key “foo” to “bar” with an expiration time of 1 minute. We then get the value of the key “foo” from the cache and print it. Finally, we delete the key “foo” from the cache.
Using a cache in Go can significantly improve the performance of web applications by reducing the number of requests to the database or external APIs. The “go-cache” library is a simple and effective caching solution that can be used in any Go application.
What is a Cache in Go?
In conclusion, a cache in Go is a powerful tool that can significantly improve the performance of your application. By storing frequently accessed data in memory, a cache can reduce the number of expensive database or network calls, resulting in faster response times and reduced latency. Go provides several built-in caching mechanisms, including the sync.Map and the expvar package, as well as third-party libraries like groupcache and bigcache. When implementing a cache in your Go application, it’s important to consider factors like cache size, eviction policies, and concurrency to ensure optimal performance. With the right approach, a cache can be a valuable asset in any Go developer’s toolkit.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |