A cache is a data structure used in computer science to store frequently accessed data in a faster and more efficient way. It is typically used to improve the performance of a system by reducing the time it takes to access data that is frequently used. The cache works by storing a copy of the data in a faster and more accessible location, such as in memory, so that it can be retrieved quickly when needed. When data is requested, the cache is checked first, and if the data is found, it is returned immediately. If the data is not found in the cache, it is retrieved from the slower storage location and added to the cache for future use. Keep reading below to learn how to use a Cache in Java.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
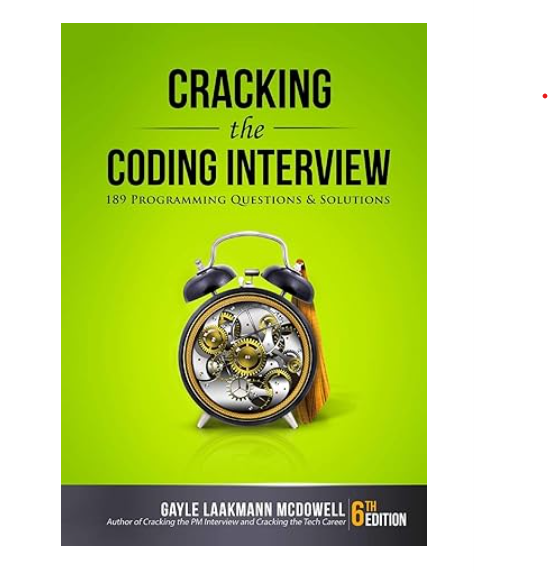
How to use a Cache in Java with example code
Caching is a technique used to store frequently accessed data in memory so that it can be retrieved quickly. In Java, caching can be implemented using various libraries such as Ehcache, Guava Cache, and Caffeine. In this blog post, we will explore how to use caching in Java with an example code using the Caffeine library.
To use Caffeine, we first need to add the following dependency to our project:
<dependency>
<groupId>com.github.ben-manes.caffeine</groupId>
<artifactId>caffeine</artifactId>
<version>2.9.0</version>
</dependency>
Once we have added the dependency, we can create a cache instance as follows:
Cache<String, String> cache = Caffeine.newBuilder()
.expireAfterWrite(5, TimeUnit.MINUTES)
.maximumSize(100)
.build();
In the above code, we have created a cache that can store up to 100 entries and will expire entries that have not been accessed for 5 minutes.
To add an entry to the cache, we can use the put method:
cache.put("key", "value");
To retrieve an entry from the cache, we can use the get method:
String value = cache.getIfPresent("key");
If the entry is not present in the cache, the get method will return null.
We can also use the invalidate method to remove an entry from the cache:
cache.invalidate("key");
In addition to the above methods, Caffeine provides various other methods to interact with the cache such as cleanUp, which can be used to remove expired entries from the cache.
In conclusion, caching is a powerful technique that can significantly improve the performance of our Java applications. Caffeine is a popular caching library that provides a simple and efficient way to implement caching in Java. By using caching, we can reduce the number of expensive operations and improve the overall performance of our applications.
What is a Cache in Java?
In conclusion, a cache in Java is a temporary storage area that stores frequently accessed data to improve the performance of an application. It works by keeping a copy of data that has been recently accessed in a faster storage location, such as memory, so that it can be quickly retrieved when needed again. Caching is an important technique used in many Java applications to reduce the amount of time it takes to access data and improve overall performance. By understanding how caching works and implementing it effectively, developers can create faster and more efficient applications that provide a better user experience.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |