A cache is a data structure used in computer science to store frequently accessed data in a faster and more efficient way. It is typically used to improve the performance of a system by reducing the time it takes to access data that is frequently used. The cache works by storing a copy of the data in a faster and more accessible location, such as in memory, so that it can be retrieved quickly when needed. When data is requested, the system first checks the cache to see if it is already stored there before accessing the original source. This helps to reduce the amount of time it takes to access the data and can significantly improve the overall performance of the system. Keep reading below to learn how to use a Cache in PHP.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
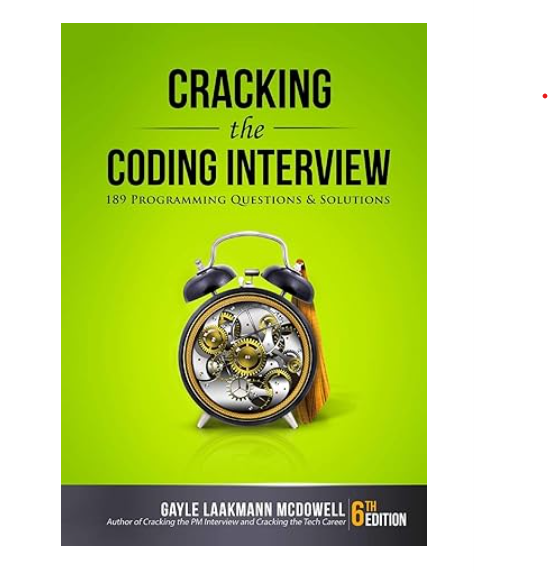
How to use a Cache in PHP with example code
Caching is an important technique used in web development to improve the performance of web applications. In PHP, caching can be implemented using various techniques such as file caching, database caching, and memory caching. In this blog post, we will discuss how to use caching in PHP with an example code.
One of the most popular caching techniques in PHP is memory caching using the Memcached extension. Memcached is a distributed memory caching system that allows you to store and retrieve data from memory. It is fast, scalable, and easy to use.
To use Memcached in PHP, you need to install the Memcached extension on your server. Once installed, you can use the following code to connect to the Memcached server:
$memcached = new Memcached();
$memcached->addServer('localhost', 11211);
In the above code, we create a new instance of the Memcached class and add a server to it. The addServer() method takes two parameters: the hostname of the Memcached server and the port number.
Once connected to the Memcached server, you can use the set() and get() methods to store and retrieve data from the cache. For example, the following code stores a string value in the cache:
$memcached->set('mykey', 'myvalue', 3600);
In the above code, we store a string value ‘myvalue’ with the key ‘mykey’ in the cache for 3600 seconds (1 hour).
To retrieve the value from the cache, you can use the get() method:
$value = $memcached->get('mykey');
In the above code, we retrieve the value of the key ‘mykey’ from the cache and store it in the $value variable.
Using caching in PHP can significantly improve the performance of your web application by reducing the number of database queries and expensive computations. However, it is important to use caching judiciously and invalidate the cache when necessary to ensure that the data is always up-to-date.
In conclusion, caching is an important technique in PHP that can help improve the performance of your web application. Memory caching using the Memcached extension is a popular and easy-to-use caching technique that can be used to store and retrieve data from memory.
What is a Cache in PHP?
In conclusion, a cache in PHP is a mechanism that stores frequently accessed data in memory or on disk to improve the performance of web applications. By reducing the number of database queries and file reads, a cache can significantly speed up the response time of a website or web application. PHP provides several caching mechanisms, including APC, Memcached, and Redis, each with its own strengths and weaknesses. When used correctly, caching can be a powerful tool for optimizing the performance of PHP applications and delivering a better user experience.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |