A cache is a data structure used in computer science to store frequently accessed data in a faster and more efficient way. It is typically used to improve the performance of a system by reducing the time it takes to access data that is frequently used. The cache works by storing a copy of the data in a faster and more accessible location, such as in memory, so that it can be retrieved quickly when needed. When data is requested, the cache is checked first, and if the data is found, it is returned immediately. If the data is not found in the cache, it is retrieved from the slower storage location and added to the cache for future use. Keep reading below to learn how to use a Cache in Python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
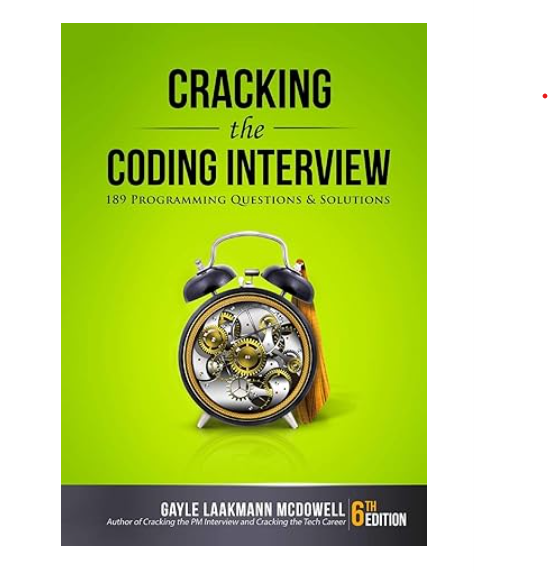
How to use a Cache in Python with example code
Caching is a technique used to store frequently accessed data in a temporary storage area so that it can be retrieved faster. In Python, caching can be implemented using various libraries such as `lru_cache`, `cachetools`, and `redis`.
One of the most commonly used caching libraries in Python is `lru_cache`. It is a built-in library that provides a simple way to cache function results. The `lru_cache` decorator can be used to cache the results of a function call.
Here is an example of how to use `lru_cache`:
import functools
@functools.lru_cache(maxsize=128)
def fibonacci(n):
if n == 0:
return 0
elif n == 1:
return 1
else:
return fibonacci(n-1) + fibonacci(n-2)
In the above example, the `fibonacci` function is decorated with `lru_cache`. The `maxsize` parameter specifies the maximum number of function calls that can be cached. If the cache is full, the least recently used result will be discarded to make room for the new result.
When the `fibonacci` function is called with a value of `n`, the result is cached. If the function is called again with the same value of `n`, the cached result is returned instead of recalculating the result.
Caching can significantly improve the performance of Python applications by reducing the time it takes to retrieve frequently accessed data. However, it is important to use caching judiciously and to monitor the cache to ensure that it is not consuming too much memory.
What is a Cache in Python?
In conclusion, a cache in Python is a temporary storage area that stores frequently accessed data to improve the performance of an application. It helps to reduce the time taken to retrieve data from the database or file system by storing it in memory. Caching is a common technique used in web development, machine learning, and other areas of software development. Python provides several caching libraries such as Flask-Cache, Django-Cache, and Redis-Cache that make it easy to implement caching in your application. By using caching, you can significantly improve the performance of your Python application and provide a better user experience.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |