A cache is a data structure used in computer science to store frequently accessed data in a faster and more efficient way. It is typically used to improve the performance of a system by reducing the time it takes to access data that is frequently used. The cache works by storing a copy of the data in a faster and more accessible location, such as in memory, so that it can be retrieved quickly when needed. When data is requested, the cache is checked first, and if the data is found, it is returned immediately. If the data is not found in the cache, it is retrieved from the slower storage location and added to the cache for future use. Keep reading below to learn how to use a Cache in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
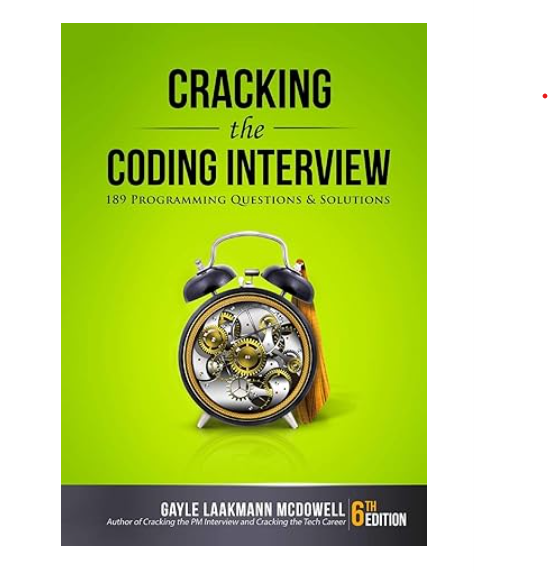
How to use a Cache in Rust with example code
Caching is an important technique used in software development to improve performance. In Rust, caching can be implemented using various libraries such as `lru-cache`, `hashbrown`, and `dashmap`. In this blog post, we will explore how to use the `lru-cache` library to implement caching in Rust.
To get started, we need to add the `lru-cache` library to our project’s dependencies in `Cargo.toml`:
[dependencies]
lru-cache = "0.1.2"
Once we have added the dependency, we can create a new cache instance using the `LruCache` struct:
use lru_cache::LruCache;
let mut cache = LruCache::new(10);
In this example, we have created a cache with a maximum capacity of 10 items. We can now insert items into the cache using the `insert` method:
cache.insert("key1", "value1");
cache.insert("key2", "value2");
We can retrieve items from the cache using the `get` method:
let value1 = cache.get(&"key1");
let value2 = cache.get(&"key2");
If an item is not present in the cache, the `get` method will return `None`. We can also remove items from the cache using the `remove` method:
cache.remove(&"key1");
Finally, we can clear the entire cache using the `clear` method:
cache.clear();
In conclusion, caching is an important technique for improving performance in software development. In Rust, we can implement caching using various libraries such as `lru-cache`. By using a cache, we can reduce the number of expensive operations and improve the overall performance of our applications.
What is a Cache in Rust?
In conclusion, a cache in Rust is a data structure that stores frequently accessed data in memory to improve the performance of an application. Caching is a common technique used in software development to reduce the time it takes to access data from slower storage devices such as hard drives or databases. Rust provides several caching libraries that developers can use to implement caching in their applications. These libraries offer different caching strategies and features that can be tailored to specific use cases. By using caching in Rust, developers can significantly improve the performance of their applications and provide a better user experience.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |