A cache is a data structure used in computer science to store frequently accessed data in a faster and more efficient way. It is typically used to improve the performance of a system by reducing the time it takes to access data that is frequently used. The cache works by storing a copy of the data in a faster and more accessible location, such as in memory, so that it can be retrieved quickly when needed. When data is requested, the cache is checked first, and if the data is found, it is returned immediately. If the data is not found in the cache, it is retrieved from the slower storage location and added to the cache for future use. Keep reading below to learn how to use a Cache in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
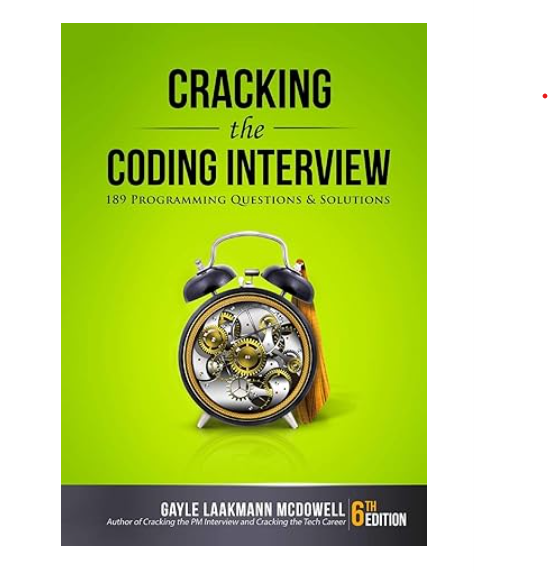
How to use a Cache in TypeScript with example code
Caching is a technique used to store frequently accessed data in memory or disk to reduce the number of requests made to the server. In TypeScript, we can use caching to improve the performance of our applications. In this blog post, we will discuss how to use a cache in TypeScript with example code.
To implement caching in TypeScript, we can use a Map object. A Map object is a collection of key-value pairs where each key is unique. We can use the key to retrieve the corresponding value from the Map object. Here is an example code that demonstrates how to use a Map object as a cache:
const cache = new Map();
function getData(key: string) {
if (cache.has(key)) {
return cache.get(key);
} else {
const data = fetchDataFromServer(key);
cache.set(key, data);
return data;
}
}
In the above code, we have created a cache object using the Map constructor. The getData function takes a key as an argument and checks if the cache object has the key. If the key exists in the cache object, the function returns the corresponding value. If the key does not exist in the cache object, the function fetches the data from the server, stores it in the cache object using the set method, and returns the data.
Using a cache can significantly improve the performance of our applications by reducing the number of requests made to the server. However, we need to be careful when using a cache as it can consume a lot of memory if not used properly. We should also consider setting an expiration time for the cached data to ensure that we are always using the latest data.
In conclusion, caching is a powerful technique that can improve the performance of our TypeScript applications. By using a Map object as a cache, we can store frequently accessed data in memory and reduce the number of requests made to the server.
What is a Cache in TypeScript?
In conclusion, a cache in TypeScript is a data structure that stores frequently accessed data in memory to improve the performance of an application. It works by storing a copy of the data in a cache, which can be quickly accessed when needed, instead of retrieving it from the original source every time. Caching is a powerful technique that can significantly improve the speed and efficiency of an application, especially when dealing with large amounts of data. By using caches, developers can reduce the load on servers, minimize network traffic, and provide a better user experience. In TypeScript, there are several caching libraries available that can be used to implement caching in an application. Overall, understanding how caching works and how to use it effectively can help developers build faster and more efficient applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |