A dictionary is a data structure in computer science that stores data in key-value pairs. Each key is unique and is used to access its corresponding value. The dictionary is implemented as a hash table, which allows for fast access and retrieval of data. It is commonly used in programming languages to store and manipulate data, such as in Python where it is represented by the dict() function. Dictionaries are useful for storing large amounts of data and for quickly accessing specific values based on their keys. Keep reading below to learn how to use a Dictionary in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
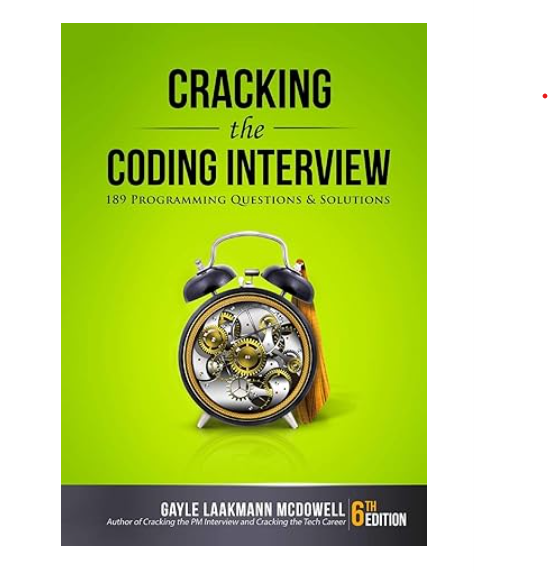
How to use a Dictionary in Rust with example code
A dictionary is a collection of key-value pairs, where each key is unique. In Rust, dictionaries are implemented using the `HashMap` data structure. To use a `HashMap`, you first need to import it from the standard library:
use std::collections::HashMap;
Once you have imported `HashMap`, you can create a new dictionary like this:
let mut my_dict = HashMap::new();
This creates a new, empty dictionary called `my_dict`. The `mut` keyword is used to make the dictionary mutable, which means you can add and remove items from it.
To add an item to the dictionary, you use the `insert` method:
my_dict.insert("key", "value");
This adds a new key-value pair to the dictionary. The first argument is the key, and the second argument is the value.
To retrieve a value from the dictionary, you use the `get` method:
let value = my_dict.get("key");
This retrieves the value associated with the key “key”. Note that the `get` method returns an `Option` type, which means it could return `Some(value)` if the key exists in the dictionary, or `None` if the key does not exist.
To remove an item from the dictionary, you use the `remove` method:
my_dict.remove("key");
This removes the key-value pair associated with the key “key” from the dictionary.
Here is an example program that demonstrates how to use a `HashMap`:
use std::collections::HashMap;
fn main() {
let mut my_dict = HashMap::new();
my_dict.insert("apple", 3);
my_dict.insert("banana", 2);
my_dict.insert("orange", 5);
let num_oranges = my_dict.get("orange");
match num_oranges {
Some(num) => println!("There are {} oranges", num),
None => println!("There are no oranges"),
}
my_dict.remove("banana");
for (key, value) in my_dict.iter() {
println!("{}: {}", key, value);
}
}
This program creates a dictionary with three key-value pairs, retrieves the value associated with the key “orange”, removes the key-value pair associated with the key “banana”, and then iterates over the remaining key-value pairs and prints them to the console.
What is a Dictionary in Rust?
In conclusion, a dictionary in Rust is a powerful data structure that allows for efficient storage and retrieval of key-value pairs. It is implemented using the HashMap data structure, which uses a hash function to map keys to their corresponding values. Rust’s dictionary implementation provides a safe and efficient way to work with collections of data, making it a popular choice for developers working on a wide range of projects. Whether you’re building a web application, a game, or a scientific simulation, Rust’s dictionary is a valuable tool that can help you manage your data with ease. So, if you’re looking for a reliable and efficient way to store and retrieve key-value pairs in your Rust project, be sure to consider using a dictionary.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |