A dictionary is a data structure in computer science that stores data in key-value pairs. Each key is unique and is used to access its corresponding value. The dictionary is implemented as an associative array, where the keys are hashed to provide fast access to the values. Dictionaries are commonly used to store and retrieve data in a way that is efficient and easy to understand. They are used in a wide range of applications, including databases, search engines, and programming languages. Keep reading below to learn how to use a Dictionary in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
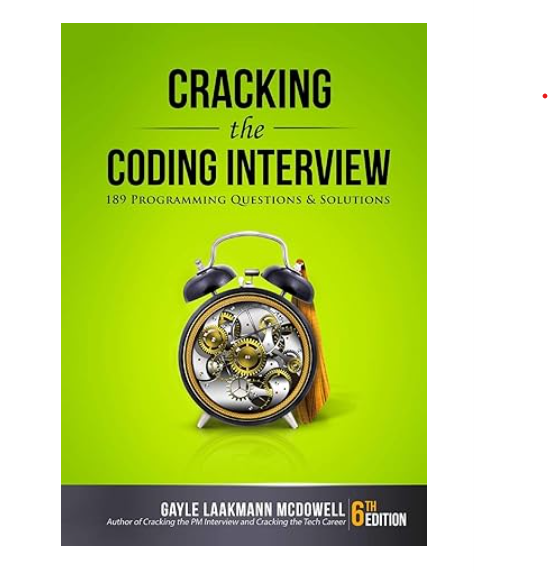
How to use a Dictionary in TypeScript with example code
A Dictionary is a collection of key-value pairs in TypeScript. It is similar to a Map in JavaScript. In this blog post, we will learn how to use a Dictionary in TypeScript with example code.
To use a Dictionary in TypeScript, we first need to import the Dictionary class from the ‘typescript-collections’ library. We can install this library using npm by running the following command:
npm install typescript-collections
Once we have installed the library, we can import the Dictionary class in our TypeScript file as follows:
import { Dictionary } from 'typescript-collections';
We can then create a new instance of the Dictionary class as follows:
const dict = new Dictionary<string, number>();
In this example, we have created a Dictionary that maps strings to numbers. We can add key-value pairs to the Dictionary using the set() method as follows:
dict.set('one', 1);
dict.set('two', 2);
We can retrieve the value associated with a key using the get() method as follows:
const value = dict.get('one');
In this example, the value variable will be set to 1.
We can check if a key exists in the Dictionary using the containsKey() method as follows:
const exists = dict.containsKey('one');
In this example, the exists variable will be set to true.
We can remove a key-value pair from the Dictionary using the remove() method as follows:
dict.remove('one');
In this example, the key-value pair with the key ‘one’ will be removed from the Dictionary.
In conclusion, a Dictionary is a useful data structure in TypeScript for storing key-value pairs. We can use the Dictionary class from the ‘typescript-collections’ library to create and manipulate Dictionaries in our TypeScript code.
What is a Dictionary in TypeScript?
In conclusion, a dictionary in TypeScript is a powerful data structure that allows developers to store and retrieve key-value pairs efficiently. It provides a flexible and dynamic way to manage data, making it an essential tool for any TypeScript project. With its ability to handle large amounts of data and its ease of use, a dictionary is a valuable asset for developers looking to create robust and scalable applications. Whether you’re building a simple web application or a complex enterprise system, a dictionary in TypeScript is a must-have tool in your development arsenal.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |