A graph is a data structure that consists of a set of vertices (also known as nodes) and a set of edges that connect these vertices. Each edge represents a relationship or connection between two vertices. Graphs can be directed, where the edges have a specific direction, or undirected, where the edges have no direction. Graphs are commonly used in computer science to model complex systems, such as social networks, transportation networks, and computer networks. They are also used in algorithms for tasks such as shortest path finding, network flow optimization, and clustering. Keep reading below to learn how to use a Graph in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
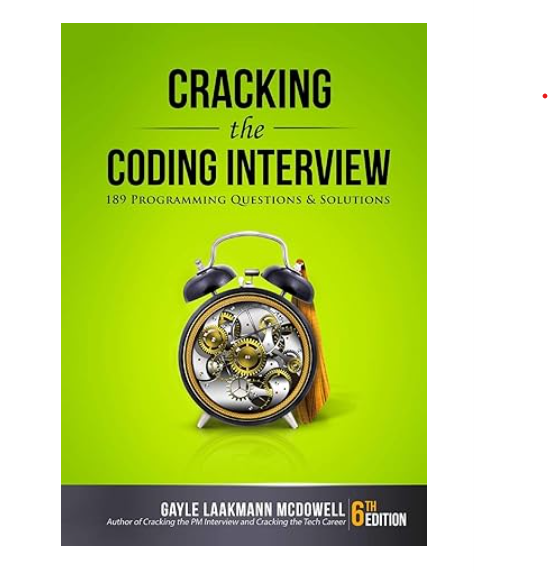
How to use a Graph in Go with example code
Graphs are a fundamental data structure in computer science and are used to model relationships between objects. In Go, graphs can be implemented using various data structures such as adjacency lists or matrices. In this blog post, we will explore how to use a graph in Go with an example code.
To begin with, let’s define a graph using an adjacency list. An adjacency list is a collection of unordered lists used to represent a finite graph. Each list describes the set of neighbors of a vertex in the graph. Here’s an example of an adjacency list for a graph with four vertices:
graph := make(map[int][]int)
graph[0] = []int{1, 2}
graph[1] = []int{2}
graph[2] = []int{0, 3}
graph[3] = []int{3}
In this example, we have a graph with four vertices (0, 1, 2, and 3). The adjacency list for vertex 0 contains vertices 1 and 2, the adjacency list for vertex 1 contains vertex 2, and so on.
Now, let’s explore how to traverse a graph using depth-first search (DFS). DFS is a recursive algorithm that visits all the vertices of a graph in a depthward motion. Here’s an example of DFS implementation in Go:
func dfs(graph map[int][]int, visited map[int]bool, vertex int) {
visited[vertex] = true
fmt.Println(vertex)
for _, v := range graph[vertex] {
if !visited[v] {
dfs(graph, visited, v)
}
}
}
In this example, we have defined a function dfs that takes three arguments: the graph, a map of visited vertices, and the starting vertex. The function marks the starting vertex as visited and prints it. Then, it recursively visits all the unvisited neighbors of the starting vertex.
Finally, let’s see how to use the graph and DFS implementation to solve a problem. Consider the problem of finding the number of connected components in a graph. A connected component is a subgraph in which any two vertices are connected to each other by a path.
func countComponents(n int, edges [][]int) int {
graph := make(map[int][]int)
visited := make(map[int]bool)
count := 0
for i := 0; i < n; i++ { visited[i] = false graph[i] = []int{} } for _, edge := range edges { u, v := edge[0], edge[1] graph[u] = append(graph[u], v) graph[v] = append(graph[v], u) } for i := 0; i < n; i++ { if !visited[i] { dfs(graph, visited, i) count++ } } return count }
In this example, we have defined a function countComponents that takes two arguments: the number of vertices in the graph and a list of edges. The function creates a graph using the adjacency list and initializes a map of visited vertices. Then, it uses DFS to traverse the graph and count the number of connected components.
In conclusion, graphs are a powerful data structure that can be used to model complex relationships between objects. Go provides various ways to implement graphs, and DFS is a useful algorithm to traverse them. We hope this blog post has helped you understand how to use a graph in Go with an example code.
What is a Graph in Go?
In conclusion, a graph in Go is a powerful data structure that allows developers to represent complex relationships between objects or entities. With its flexible and efficient implementation, Go's graph package provides a range of algorithms and functions that enable developers to manipulate and analyze graphs with ease. Whether you're building a social network, a recommendation engine, or a routing system, understanding the basics of graph theory and how to use graphs in Go can help you create more robust and scalable applications. So, if you're looking to take your programming skills to the next level, learning about graphs in Go is definitely worth your time and effort.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |