A graph is a data structure that consists of a set of vertices (also known as nodes) and a set of edges that connect these vertices. Each edge represents a relationship or connection between two vertices. Graphs can be directed, where the edges have a specific direction, or undirected, where the edges have no direction. Graphs are commonly used in computer science to model complex systems, such as social networks, transportation networks, and computer networks. They are also used in algorithms such as Dijkstra’s algorithm and Kruskal’s algorithm for finding the shortest path and minimum spanning tree, respectively. Keep reading below to learn how to use a Graph in Python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
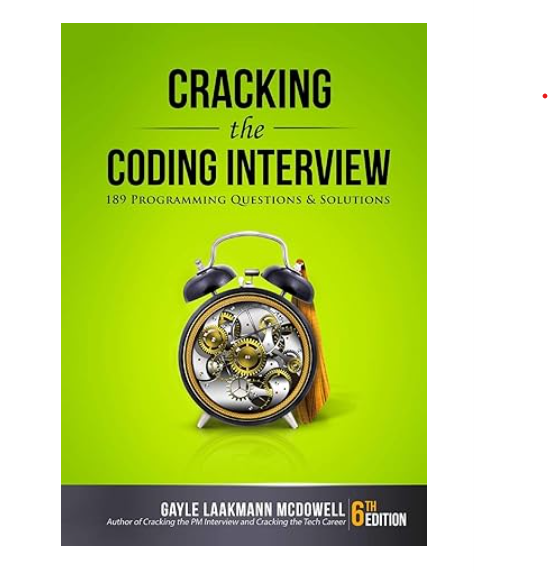
How to use a Graph in Python with example code
Python is a popular programming language used for a variety of tasks, including data analysis and visualization. One powerful tool for data visualization is the graph, which can be created using Python’s matplotlib library.
To get started with creating a graph in Python, you’ll first need to install matplotlib. This can be done using pip, the package installer for Python:
pip install matplotlib
Once you have matplotlib installed, you can begin creating your graph. The first step is to import the necessary modules:
import matplotlib.pyplot as plt
import numpy as np
Next, you’ll need to create some data to plot. For this example, we’ll create a simple line graph using the sine function:
x = np.linspace(0, 10, 100)
y = np.sin(x)
Now that we have our data, we can create the graph using the plot function:
plt.plot(x, y)
Finally, we’ll add some labels and a title to our graph:
plt.xlabel('x')
plt.ylabel('y')
plt.title('Sine Function')
And that’s it! You can now display your graph using the show function:
plt.show()
This is just a simple example, but matplotlib offers a wide range of customization options for creating more complex graphs. With a little practice, you’ll be able to create beautiful and informative visualizations of your data in no time.
What is a Graph in Python?
In conclusion, a graph in Python is a powerful data structure that allows us to represent and analyze complex relationships between objects. With the help of various Python libraries such as NetworkX and Matplotlib, we can easily create, manipulate, and visualize graphs to gain insights into our data. Whether it’s analyzing social networks, predicting traffic patterns, or optimizing supply chains, graphs are an essential tool for data scientists and programmers alike. By understanding the basics of graph theory and mastering the Python programming language, we can unlock the full potential of this versatile data structure and solve a wide range of real-world problems.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |