A graph is a data structure that consists of a set of vertices (also known as nodes) and a set of edges that connect these vertices. Each edge represents a relationship or connection between two vertices. Graphs can be directed, where the edges have a specific direction, or undirected, where the edges have no direction. Graphs are commonly used in computer science to model complex systems, such as social networks, transportation networks, and computer networks. They are also used in algorithms for tasks such as shortest path finding, network flow optimization, and clustering. Keep reading below to learn how to use a Graph in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
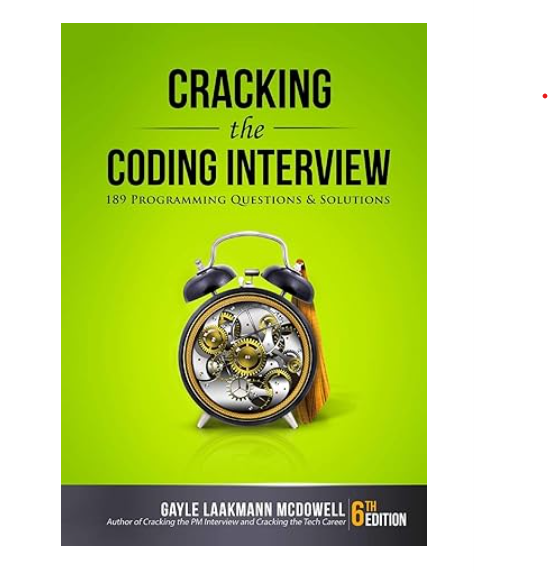
How to use a Graph in Rust with example code
Rust is a systems programming language that has been gaining popularity in recent years. One of the many benefits of Rust is its ability to work with graphs efficiently. In this blog post, we will explore how to use a graph in Rust with example code.
First, we need to understand what a graph is. A graph is a collection of nodes and edges that connect those nodes. In Rust, we can represent a graph using a struct that contains a vector of nodes and a vector of edges. Here is an example:
struct Graph {
nodes: Vec
edges: Vec
}
struct Node {
id: i32,
}
struct Edge {
from: i32,
to: i32,
}
In this example, we have a graph that contains nodes and edges. Each node has an ID, and each edge has a “from” and “to” node ID. We can use this struct to represent any type of graph.
Now that we have our graph struct, we can start adding nodes and edges to it. Here is an example of how to add a node to the graph:
let mut graph = Graph {
nodes: Vec::new(),
edges: Vec::new(),
};
let node = Node { id: 1 };
graph.nodes.push(node);
In this example, we create a new graph and add a node with an ID of 1 to it. We use the “push” method to add the node to the vector of nodes.
Next, let’s add an edge to the graph. Here is an example:
let mut graph = Graph {
nodes: Vec::new(),
edges: Vec::new(),
};
let node1 = Node { id: 1 };
let node2 = Node { id: 2 };
graph.nodes.push(node1);
graph.nodes.push(node2);
let edge = Edge { from: 1, to: 2 };
graph.edges.push(edge);
In this example, we create two nodes with IDs of 1 and 2, add them to the graph, and then add an edge that connects node 1 to node 2.
Finally, we can traverse the graph using various algorithms such as depth-first search or breadth-first search. Here is an example of how to perform a depth-first search on the graph:
fn dfs(graph: &Graph, node_id: i32, visited: &mut Vec
visited[node_id as usize] = true;
println!("Visited node {}", node_id);
for edge in &graph.edges {
if edge.from == node_id && !visited[edge.to as usize] {
dfs(graph, edge.to, visited);
}
}
}
let mut visited = vec![false; graph.nodes.len()];
dfs(&graph, 1, &mut visited);
In this example, we define a function called “dfs” that performs a depth-first search on the graph. We start at a given node ID, mark it as visited, and then recursively visit all of its neighbors. We use a vector called “visited” to keep track of which nodes we have already visited.
In conclusion, Rust is a powerful language for working with graphs. With its efficient memory management and strong type system, Rust is an excellent choice for building graph-based applications. By using the graph struct and various algorithms, we can easily create and traverse graphs in Rust.
What is a Graph in Rust?
In conclusion, a graph is a powerful data structure that can be used to represent complex relationships between objects. In Rust, there are several libraries available that provide efficient and easy-to-use implementations of graphs. These libraries offer a range of features, from simple graph representations to more advanced algorithms for graph traversal and manipulation. Whether you are working on a small project or a large-scale application, understanding how to use graphs in Rust can help you to build more efficient and effective software. So, if you are looking to take your Rust programming skills to the next level, learning about graphs is definitely worth your time and effort.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |