A Hash Table is a data structure that stores data in an associative array format, where each data element is assigned a unique key. The key is then used to access the data in constant time, making it a very efficient data structure for searching, inserting, and deleting data. The Hash Table uses a hash function to map the key to an index in an array, where the data is stored. In case of collisions, where two keys map to the same index, the Hash Table uses a collision resolution technique to handle the situation. Overall, the Hash Table is a widely used data structure in computer science due to its efficiency and versatility. Keep reading below to learn how to use a Hash Table in Bash.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
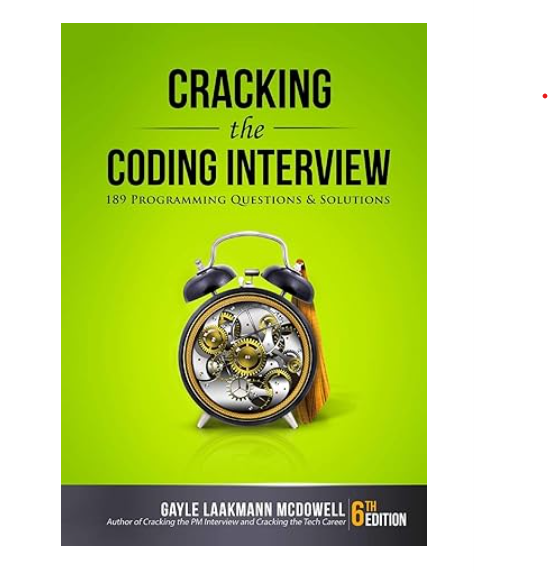
How to use a Hash Table in Bash with example code
Hash tables are a useful data structure in Bash that allow you to store and retrieve key-value pairs quickly. In this blog post, we will explore how to use a hash table in Bash with example code.
To create a hash table in Bash, you can use the `declare` command with the `-A` option. Here’s an example:
declare -A my_hash
This creates an empty hash table called `my_hash`. You can then add key-value pairs to the hash table using the following syntax:
my_hash[key]=value
For example, let’s add some key-value pairs to `my_hash`:
my_hash["apple"]="red"
my_hash["banana"]="yellow"
my_hash["grape"]="purple"
To retrieve the value associated with a key, you can use the following syntax:
echo ${my_hash[key]}
For example, to retrieve the color of an apple, you can use:
echo ${my_hash["apple"]}
This will output `red`.
You can also loop through all the keys in a hash table using a `for` loop. Here’s an example:
for key in "${!my_hash[@]}"
do
echo "The color of $key is ${my_hash[$key]}"
done
This will output:
The color of apple is red
The color of banana is yellow
The color of grape is purple
In conclusion, hash tables are a powerful data structure in Bash that allow you to store and retrieve key-value pairs quickly. By using the `declare` command with the `-A` option, you can create a hash table in Bash. You can add key-value pairs to the hash table using the syntax `my_hash[key]=value`, and retrieve the value associated with a key using the syntax `${my_hash[key]}`. You can also loop through all the keys in a hash table using a `for` loop.
What is a Hash Table in Bash?
In conclusion, a Hash Table in Bash is a powerful data structure that allows for efficient storage and retrieval of key-value pairs. It works by using a hash function to map keys to indices in an array, which allows for constant-time access to values. Hash Tables are commonly used in programming for tasks such as caching, indexing, and searching. By understanding the basics of Hash Tables in Bash, developers can improve the performance and efficiency of their scripts and applications. Overall, Hash Tables are an essential tool in any programmer’s toolkit, and mastering them can lead to more efficient and effective code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |