A Hash Table is a data structure that stores data in an associative array format, where each data element is assigned a unique key. The key is then used to access the data in constant time, making it a very efficient data structure for searching, inserting, and deleting data. The Hash Table uses a hash function to map the key to an index in an array, where the data is stored. The hash function ensures that each key is mapped to a unique index, and collisions are handled by using a collision resolution technique such as chaining or open addressing. The Hash Table is widely used in computer science for implementing databases, caches, and other data-intensive applications. Keep reading below to learn how to use a Hash Table in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
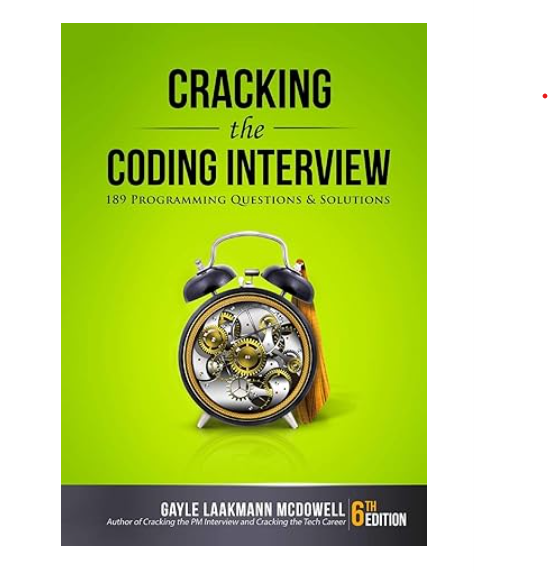
How to use a Hash Table in C# with example code
Hash tables are a fundamental data structure in computer science that allow for efficient storage and retrieval of key-value pairs. In C#, hash tables are implemented using the `Dictionary
To use a hash table in C#, you first need to create an instance of the `Dictionary
“`csharp
Dictionary
“`
In this example, we are creating a dictionary that maps strings to integers. Once you have created a dictionary, you can add key-value pairs to it using the `Add` method.
“`csharp
myDictionary.Add(“one”, 1);
myDictionary.Add(“two”, 2);
myDictionary.Add(“three”, 3);
“`
In this example, we are adding three key-value pairs to the dictionary. The first key is “one” and the corresponding value is 1. The second key is “two” and the corresponding value is 2. The third key is “three” and the corresponding value is 3.
To retrieve a value from the dictionary, you can use the indexer syntax.
“`csharp
int value = myDictionary[“two”];
“`
In this example, we are retrieving the value associated with the key “two”. The variable `value` will be set to 2.
You can also check if a key exists in the dictionary using the `ContainsKey` method.
“`csharp
if (myDictionary.ContainsKey(“four”))
{
Console.WriteLine(“The dictionary contains the key ‘four'”);
}
else
{
Console.WriteLine(“The dictionary does not contain the key ‘four'”);
}
“`
In this example, we are checking if the dictionary contains the key “four”. Since we did not add a key-value pair with the key “four”, the output will be “The dictionary does not contain the key ‘four'”.
Finally, you can iterate over the key-value pairs in the dictionary using a `foreach` loop.
“`csharp
foreach (KeyValuePair
{
Console.WriteLine(“Key = {0}, Value = {1}”, kvp.Key, kvp.Value);
}
“`
In this example, we are iterating over the key-value pairs in the dictionary and printing out each key-value pair. The output will be:
“`
Key = one, Value = 1
Key = two, Value = 2
Key = three, Value = 3
“`
In conclusion, hash tables are a powerful data structure that allow for efficient storage and retrieval of key-value pairs. In C#, hash tables are implemented using the `Dictionary
What is a Hash Table in C#?
In conclusion, a Hash Table in C# is a powerful data structure that allows for efficient storage and retrieval of key-value pairs. It works by using a hash function to map keys to specific indexes in an array, making it easy to locate and access values. Hash Tables are commonly used in a variety of applications, including databases, search engines, and caching systems. With its fast performance and flexible design, a Hash Table is an essential tool for any developer working with large amounts of data in C#.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |