A Hash Table is a data structure that allows for efficient storage and retrieval of key-value pairs. It works by using a hash function to map each key to a unique index in an array. When a value is inserted into the hash table, its key is hashed to determine its index in the array. If there is already a value stored at that index, a collision occurs and the hash table uses a collision resolution strategy to handle it. Common collision resolution strategies include chaining and open addressing. Hash tables have an average time complexity of O(1) for insertion, deletion, and retrieval operations, making them a popular choice for implementing dictionaries and symbol tables. Keep reading below to learn how to use a Hash Table in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
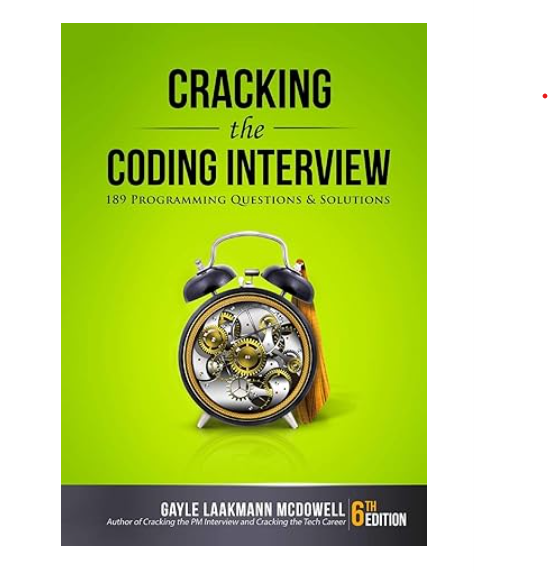
How to use a Hash Table in Go with example code
Hash tables, also known as hash maps, are a powerful data structure used to store key-value pairs. In Go, hash tables are implemented using the built-in `map` type. In this blog post, we will explore how to use hash tables in Go with an example code.
To create a hash table in Go, we use the `make` function with the `map` keyword. Here’s an example:
ages := make(map[string]int)
In this example, we create a hash table called `ages` that maps strings to integers. We can add key-value pairs to the hash table using the following syntax:
ages["Alice"] = 31
This adds a key-value pair to the `ages` hash table, where the key is `”Alice”` and the value is `31`. We can retrieve the value associated with a key using the following syntax:
fmt.Println(ages["Alice"])
This will print `31` to the console.
We can also check if a key exists in the hash table using the following syntax:
age, ok := ages["Bob"]
In this example, we check if the key `”Bob”` exists in the `ages` hash table. If it does, the value associated with the key is assigned to the `age` variable and `ok` is set to `true`. If the key does not exist, `ok` is set to `false`.
We can iterate over all the key-value pairs in a hash table using a `for` loop with the `range` keyword. Here’s an example:
for name, age := range ages {
fmt.Printf("%s is %d years old\n", name, age)
}
In this example, we iterate over all the key-value pairs in the `ages` hash table and print out each person’s name and age.
Hash tables are a powerful data structure that can be used to efficiently store and retrieve key-value pairs. In Go, hash tables are implemented using the built-in `map` type. By using hash tables, we can write more efficient and concise code.
What is a Hash Table in Go?
In conclusion, a Hash Table is a powerful data structure that allows for efficient storage and retrieval of key-value pairs. In Go, Hash Tables are implemented using the built-in map data type, which provides a simple and intuitive interface for working with Hash Tables. By using a Hash Table, developers can improve the performance of their applications by reducing the time it takes to search for and retrieve data. Additionally, Hash Tables can be used in a wide range of applications, from simple data storage to complex algorithms and data analysis. Overall, understanding how to use Hash Tables in Go is an important skill for any developer looking to build efficient and scalable applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |