A Hash Table is a data structure that stores data in an associative array format, where each data element is assigned a unique key. The key is then used to access the data in constant time, making it a very efficient data structure for searching, inserting, and deleting data. The Hash Table uses a hash function to map the key to an index in an array, where the data is stored. In case of collisions, where two keys map to the same index, the Hash Table uses a collision resolution technique to handle the situation. Overall, the Hash Table is a widely used data structure in computer science due to its efficiency and versatility. Keep reading below to learn how to use a Hash Table in Javascript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
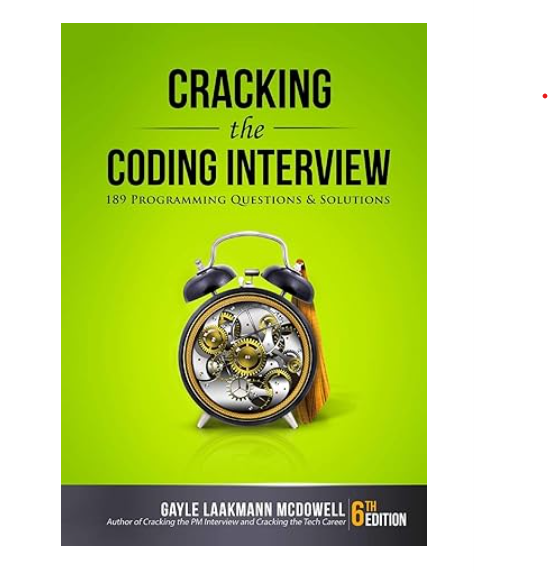
How to use a Hash Table in Javascript with example code
Hash tables, also known as hash maps, are a type of data structure that allow for efficient lookup, insertion, and deletion of key-value pairs. In JavaScript, hash tables can be implemented using objects.
To create a hash table in JavaScript, simply create a new object and add key-value pairs to it using the following syntax:
let hashTable = {};
hashTable[key] = value;
To retrieve a value from the hash table, simply access it using the key:
let value = hashTable[key];
To delete a key-value pair from the hash table, use the delete
keyword:
delete hashTable[key];
Here’s an example of a hash table in action:
// Create a new hash table
let colors = {};
// Add some key-value pairs
colors["red"] = "#FF0000";
colors["green"] = "#00FF00";
colors["blue"] = "#0000FF";
// Retrieve a value
let redValue = colors["red"];
console.log(redValue); // Output: "#FF0000"
// Delete a key-value pair
delete colors["green"];
console.log(colors); // Output: { "red": "#FF0000", "blue": "#0000FF" }
Hash tables are a powerful tool for organizing and accessing data in JavaScript. By using objects as hash tables, you can easily store and retrieve key-value pairs with minimal overhead.
What is a Hash Table in Javascript?
In conclusion, a Hash Table is a powerful data structure in Javascript that allows for efficient storage and retrieval of key-value pairs. It works by using a hash function to map keys to specific indexes in an array, making it easy to access values without having to search through the entire data structure. Hash Tables are commonly used in a variety of applications, including databases, caching systems, and search algorithms. By understanding how Hash Tables work and how to implement them in Javascript, developers can create more efficient and effective programs that can handle large amounts of data with ease.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |