A Hash Table is a data structure that stores data in an associative array format, where each data element is assigned a unique key. The key is then used to access the data in constant time, making it a very efficient data structure for searching, inserting, and deleting data. The Hash Table uses a hash function to map the key to an index in an array, where the data is stored. The hash function ensures that each key is mapped to a unique index, and collisions are handled by using a collision resolution technique such as chaining or open addressing. The Hash Table is widely used in computer science for implementing databases, caches, and other data-intensive applications. Keep reading below to learn how to use a Hash Table in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
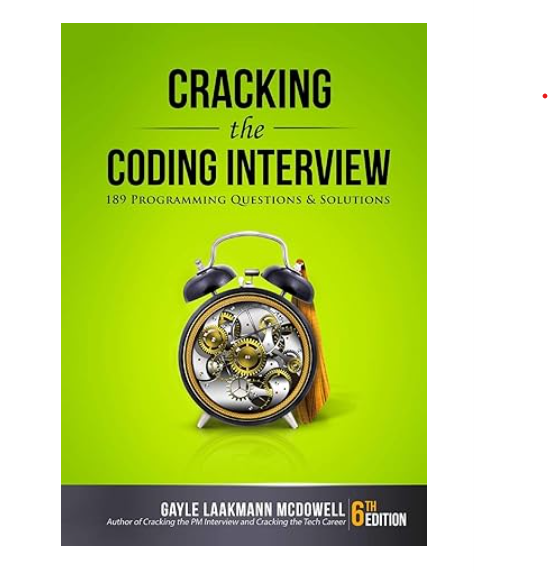
How to use a Hash Table in Kotlin with example code
Hash tables, also known as hash maps, are a popular data structure used in programming. They allow for efficient storage and retrieval of key-value pairs. In Kotlin, hash tables are implemented using the `HashMap` class.
To use a hash table in Kotlin, you first need to create a new instance of the `HashMap` class. You can do this using the following code:
val hashMap = HashMap<String, Int>()
This creates a new hash table that maps strings to integers. You can replace `String` and `Int` with any other data types you want to use.
To add a new key-value pair to the hash table, you can use the `put` method. For example:
hashMap.put("one", 1)
hashMap.put("two", 2)
hashMap.put("three", 3)
This adds three new key-value pairs to the hash table. The keys are strings (“one”, “two”, and “three”), and the values are integers (1, 2, and 3).
To retrieve a value from the hash table, you can use the `get` method. For example:
val value = hashMap.get("two")
println(value) // Output: 2
This retrieves the value associated with the key “two” from the hash table and stores it in the `value` variable. The `println` statement then prints the value to the console.
You can also check if a key exists in the hash table using the `containsKey` method. For example:
if (hashMap.containsKey("one")) {
println("The hash table contains the key 'one'")
} else {
println("The hash table does not contain the key 'one'")
}
This checks if the key “one” exists in the hash table and prints a message to the console accordingly.
Overall, hash tables are a powerful data structure that can be used in a variety of programming tasks. By using the `HashMap` class in Kotlin, you can easily create and manipulate hash tables in your code.
What is a Hash Table in Kotlin?
In conclusion, a Hash Table is a data structure that allows for efficient storage and retrieval of key-value pairs. In Kotlin, Hash Tables are implemented using the HashMap class, which provides a simple and intuitive interface for working with this data structure. By using a hashing function to map keys to indices in an array, Hash Tables can achieve constant-time complexity for both insertion and retrieval operations, making them a powerful tool for a wide range of applications. Whether you’re building a simple application or a complex system, understanding how Hash Tables work in Kotlin can help you write more efficient and effective code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |