A Hash Table is a data structure that stores data in an associative array format, where each data element is assigned a unique key. The key is then used to access the data in constant time, making it a very efficient data structure for searching, inserting, and deleting data. The Hash Table uses a hash function to map the key to an index in an array, where the data is stored. The hash function ensures that each key is mapped to a unique index, and collisions are handled by using a collision resolution technique such as chaining or open addressing. The Hash Table is widely used in computer science for implementing databases, caches, and other data-intensive applications. Keep reading below to learn how to use a Hash Table in Python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
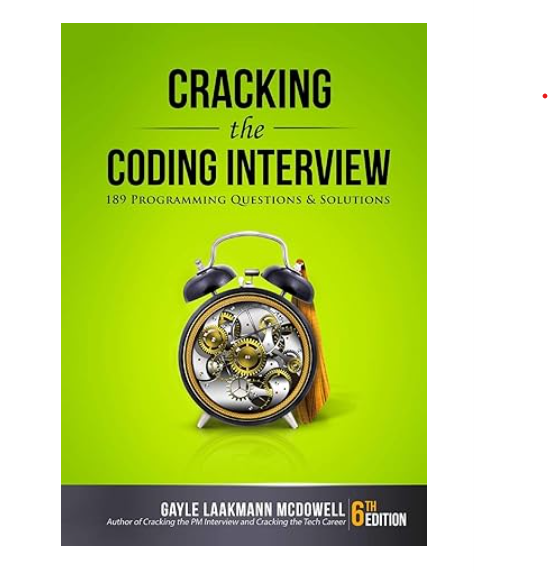
How to use a Hash Table in Python with example code
Hash tables are a fundamental data structure in computer science that allow for efficient storage and retrieval of key-value pairs. In Python, hash tables are implemented using dictionaries. In this blog post, we will explore how to use a hash table in Python with example code.
To create a dictionary in Python, we use curly braces {} and separate the key-value pairs with a colon. For example, let’s create a dictionary that maps names to ages:
ages = {'Alice': 25, 'Bob': 30, 'Charlie': 35}
To access the value associated with a key in a dictionary, we use square brackets [] and the key. For example, to get the age of Alice, we would write:
print(ages['Alice']) # Output: 25
If we try to access a key that does not exist in the dictionary, we will get a KeyError. To avoid this, we can use the get() method, which returns None if the key is not found:
print(ages.get('David')) # Output: None
We can also specify a default value to return if the key is not found:
print(ages.get('David', 'Unknown')) # Output: Unknown
To add a new key-value pair to a dictionary, we simply assign a value to a new key:
ages['David'] = 40
print(ages) # Output: {'Alice': 25, 'Bob': 30, 'Charlie': 35, 'David': 40}
To remove a key-value pair from a dictionary, we use the del keyword:
del ages['Charlie']
print(ages) # Output: {'Alice': 25, 'Bob': 30, 'David': 40}
In addition to strings and integers, we can use any immutable object as a key in a dictionary. This includes tuples, which can be useful for storing multiple values as a key. For example, let’s create a dictionary that maps (x, y) coordinates to colors:
colors = {(0, 0): 'red', (0, 1): 'green', (1, 0): 'blue', (1, 1): 'yellow'}
print(colors[(0, 1)]) # Output: green
In conclusion, hash tables are a powerful data structure that allow for efficient storage and retrieval of key-value pairs. In Python, hash tables are implemented using dictionaries, which are easy to use and versatile. By understanding how to use hash tables in Python, you can write more efficient and effective code.
What is a Hash Table in Python?
In conclusion, a hash table is a powerful data structure in Python that allows for efficient storage and retrieval of key-value pairs. It works by using a hash function to map keys to indices in an array, allowing for constant-time access to values. Hash tables are commonly used in a variety of applications, including database indexing, caching, and searching. Understanding how to implement and use hash tables in Python can greatly improve the performance and efficiency of your code. With its simplicity and effectiveness, the hash table is a valuable tool for any Python developer to have in their arsenal.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |