A Heap is a specialized tree-based data structure that is used to efficiently manage and organize a collection of elements. It is a complete binary tree where each node has a value greater than or equal to its children (in a max heap) or less than or equal to its children (in a min heap). The root node of the heap always contains the highest (or lowest) value in the heap, making it useful for implementing priority queues and sorting algorithms. Heaps can be implemented using arrays or linked lists and have a time complexity of O(log n) for insertion, deletion, and search operations. Keep reading below to learn how to use a Heap in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
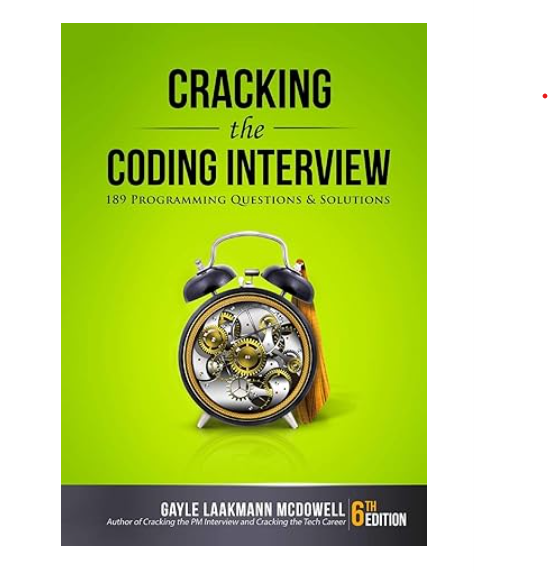
How to use a Heap in C# with example code
A heap is a data structure that is used to efficiently manage and retrieve the minimum or maximum element in a collection. In C#, heaps are implemented using arrays or lists. In this blog post, we will discuss how to use a heap in C# with example code.
To use a heap in C#, we need to first create a class that represents the heap. This class should have a constructor that initializes the heap with an empty array or list. We also need to define methods for adding elements to the heap, removing elements from the heap, and retrieving the minimum or maximum element in the heap.
Let’s take a look at an example implementation of a heap in C#:
public class MinHeap
{
private List
public MinHeap()
{
heap = new List
}
public void Add(int value)
{
heap.Add(value);
int currentIndex = heap.Count - 1;
int parentIndex = (currentIndex - 1) / 2;
while (currentIndex > 0 && heap[currentIndex] < heap[parentIndex]) { int temp = heap[currentIndex]; heap[currentIndex] = heap[parentIndex]; heap[parentIndex] = temp; currentIndex = parentIndex; parentIndex = (currentIndex - 1) / 2; } } public int RemoveMin() { if (heap.Count == 0) { throw new InvalidOperationException("Heap is empty"); } int min = heap[0]; heap[0] = heap[heap.Count - 1]; heap.RemoveAt(heap.Count - 1); int currentIndex = 0; int leftChildIndex = 2 * currentIndex + 1; int rightChildIndex = 2 * currentIndex + 2; while (leftChildIndex < heap.Count) { int smallerChildIndex = leftChildIndex; if (rightChildIndex < heap.Count && heap[rightChildIndex] < heap[leftChildIndex]) { smallerChildIndex = rightChildIndex; } if (heap[currentIndex] < heap[smallerChildIndex]) { break; } int temp = heap[currentIndex]; heap[currentIndex] = heap[smallerChildIndex]; heap[smallerChildIndex] = temp; currentIndex = smallerChildIndex; leftChildIndex = 2 * currentIndex + 1; rightChildIndex = 2 * currentIndex + 2; } return min; } public int GetMin() { if (heap.Count == 0) { throw new InvalidOperationException("Heap is empty"); } return heap[0]; } }
In this example, we have implemented a min heap using a list. The Add method adds an element to the heap and maintains the heap property by swapping elements as necessary. The RemoveMin method removes the minimum element from the heap and maintains the heap property by swapping elements as necessary. The GetMin method returns the minimum element in the heap without removing it.
To use this heap, we can create an instance of the MinHeap class and call its methods:
MinHeap heap = new MinHeap();
heap.Add(5);
heap.Add(3);
heap.Add(7);
heap.Add(1);
Console.WriteLine(heap.GetMin()); // Output: 1
heap.RemoveMin();
Console.WriteLine(heap.GetMin()); // Output: 3
In this example, we have created a min heap and added four elements to it. We then retrieved the minimum element using the GetMin method and removed the minimum element using the RemoveMin method.
What is a Heap in C#?
In conclusion, a Heap in C# is a data structure that allows for efficient storage and retrieval of data. It is a binary tree-based structure that is commonly used in computer science and programming. Heaps are particularly useful for sorting and prioritizing data, and they are commonly used in algorithms such as Dijkstra's shortest path algorithm and the A* search algorithm. Understanding how to use and implement heaps in C# can greatly improve the efficiency and effectiveness of your programming projects. By using heaps, you can optimize your code and improve the performance of your applications. Overall, a Heap in C# is a powerful tool that every programmer should have in their toolkit.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |