A Heap is a specialized tree-based data structure that is used to efficiently manage and organize a collection of elements. It is a complete binary tree where each node has a value greater than or equal to its children (in a max heap) or less than or equal to its children (in a min heap). The root node of the heap always contains the highest (or lowest) value in the heap, making it useful for implementing priority queues and sorting algorithms. Heaps can be implemented using arrays or linked lists and have a time complexity of O(log n) for insertion, deletion, and search operations. Keep reading below to learn how to use a Heap in Java.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
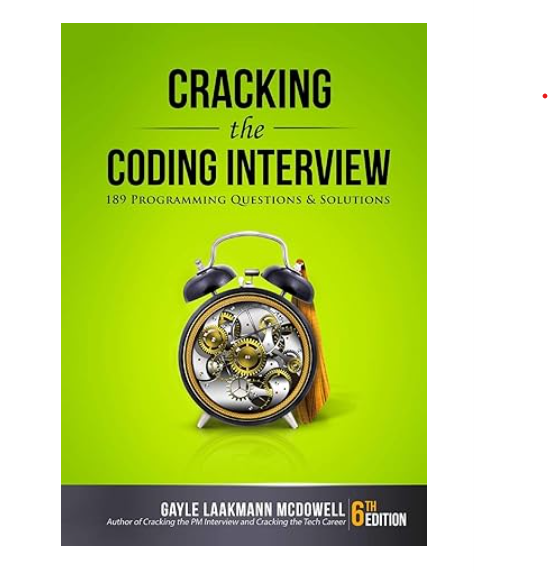
How to use a Heap in Java with example code
A Heap is a data structure that is used to represent a binary tree. It is a complete binary tree, which means that all levels of the tree are completely filled, except possibly the last level, which is filled from left to right. In Java, a Heap can be implemented using an array.
To use a Heap in Java, you first need to create an instance of the Heap class. You can do this by importing the java.util package and using the PriorityQueue class, which is a built-in implementation of a Heap in Java.
Here is an example code snippet that demonstrates how to use a Heap in Java:
import java.util.PriorityQueue;
public class HeapExample {
public static void main(String[] args) {
// Create a new PriorityQueue
PriorityQueue
// Add elements to the Heap
heap.add(3);
heap.add(1);
heap.add(5);
heap.add(2);
heap.add(4);
// Print the elements of the Heap
while (!heap.isEmpty()) {
System.out.println(heap.poll());
}
}
}
In this example, we create a new PriorityQueue and add some elements to it. We then use the poll() method to remove and print the elements of the Heap in sorted order.
Using a Heap in Java can be very useful for solving problems that involve sorting or finding the minimum or maximum element in a collection of elements.
What is a Heap in Java?
In conclusion, a Heap in Java is a data structure that allows for efficient storage and retrieval of elements based on their priority. It is a binary tree structure where each node has a value that is greater than or equal to its children. The Heap is commonly used in sorting algorithms, such as heapsort, and in priority queue implementations. Java provides built-in classes for implementing Heaps, such as the PriorityQueue class. Understanding the concept of Heaps and how to use them can greatly improve the efficiency and performance of your Java programs.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |