A Heap is a specialized tree-based data structure that is used to efficiently manage and organize a collection of elements. It is a complete binary tree where each node has a value greater than or equal to its children (in a max heap) or less than or equal to its children (in a min heap). The root node of the heap always contains the highest (in a max heap) or lowest (in a min heap) value in the heap. Heaps are commonly used in algorithms such as sorting, graph algorithms, and priority queues. They offer fast insertion and deletion of elements, as well as efficient access to the highest or lowest value in the collection. Keep reading below to learn how to use a Heap in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
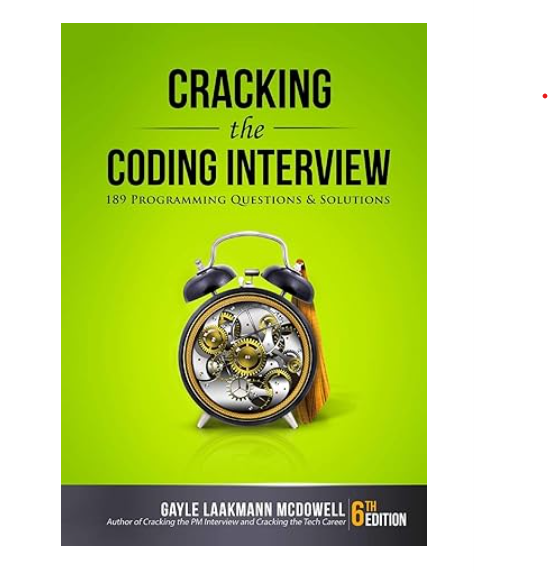
How to use a Heap in Kotlin with example code
A heap is a data structure that is used to represent a binary tree. It is a complete binary tree, which means that all levels of the tree are completely filled, except possibly the last level, which is filled from left to right. In Kotlin, heaps can be implemented using arrays.
To use a heap in Kotlin, you first need to create an array to represent the heap. The first element of the array is the root of the heap, and each subsequent element represents a node in the tree. The left child of a node at index i is located at index 2i+1, and the right child is located at index 2i+2.
Here is an example of how to create a heap in Kotlin:
class Heap {
private val heapArray = mutableListOf
fun insert(value: Int) {
heapArray.add(value)
var currentIndex = heapArray.size - 1
var parentIndex = (currentIndex - 1) / 2
while (currentIndex > 0 && heapArray[currentIndex] > heapArray[parentIndex]) {
val temp = heapArray[currentIndex]
heapArray[currentIndex] = heapArray[parentIndex]
heapArray[parentIndex] = temp
currentIndex = parentIndex
parentIndex = (currentIndex - 1) / 2
}
}
fun remove(): Int? {
if (heapArray.isEmpty()) {
return null
}
val root = heapArray[0]
heapArray[0] = heapArray[heapArray.size - 1]
heapArray.removeAt(heapArray.size - 1)
var currentIndex = 0
var childIndex = 1
while (childIndex < heapArray.size) {
if (childIndex + 1 < heapArray.size && heapArray[childIndex + 1] > heapArray[childIndex]) {
childIndex++
}
if (heapArray[currentIndex] < heapArray[childIndex]) {
val temp = heapArray[currentIndex]
heapArray[currentIndex] = heapArray[childIndex]
heapArray[childIndex] = temp
currentIndex = childIndex
childIndex = 2 * currentIndex + 1
} else {
break
}
}
return root
}
}
In this example, the Heap class has two methods: insert and remove. The insert method adds a new value to the heap and ensures that the heap property is maintained. The remove method removes the root of the heap (which is the maximum value in a max heap) and ensures that the heap property is maintained.
To use the Heap class, you can create a new instance and call the insert and remove methods as needed:
val heap = Heap()
heap.insert(5)
heap.insert(3)
heap.insert(8)
heap.insert(1)
println(heap.remove()) // prints 8
println(heap.remove()) // prints 5
In this example, the heap is initialized with four values, and the remove method is called twice to remove the two largest values from the heap.
What is a Heap in Kotlin?
In conclusion, a Heap is a data structure that is used to efficiently manage and organize data in a specific way. In Kotlin, Heaps can be implemented using the PriorityQueue class, which provides a simple and easy-to-use interface for working with Heaps. By using a Heap, developers can improve the performance of their applications by reducing the time and resources required to manage and access data. Whether you are working on a small project or a large-scale application, understanding the basics of Heaps in Kotlin can help you write more efficient and effective code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |