A Heap is a specialized tree-based data structure that is used to efficiently manage and organize a collection of elements. It is a complete binary tree where each node has a value greater than or equal to its children (in a max heap) or less than or equal to its children (in a min heap). The root node of the heap always contains the highest (or lowest) value in the heap, making it useful for implementing priority queues and sorting algorithms. Heaps can be implemented using arrays or linked lists and have a time complexity of O(log n) for insertion, deletion, and search operations. Keep reading below to learn how to use a Heap in Python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
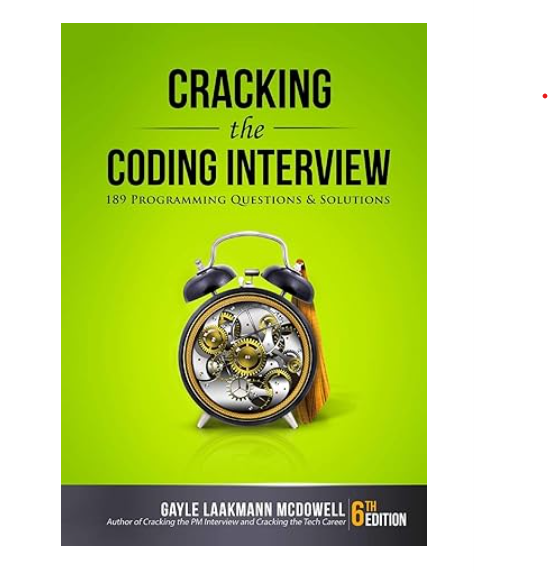
How to use a Heap in Python with example code
A heap is a data structure that is used to represent a binary tree. It is a complete binary tree, which means that all levels of the tree are completely filled, except possibly the last level, which is filled from left to right. In Python, heaps are implemented using the heapq module.
To use a heap in Python, you first need to import the heapq module. Once you have imported the module, you can create a heap by using the heapify() function. This function takes a list as an argument and converts it into a heap.
Here is an example of how to create a heap in Python:
import heapq
# create a list of numbers
numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
# convert the list into a heap
heapq.heapify(numbers)
# print the heap
print(numbers)
This will output the following:
[1, 1, 2, 3, 3, 9, 4, 6, 5, 5, 5]
As you can see, the list has been converted into a heap. The smallest element in the heap is at the root of the tree, which is the first element in the list.
You can also add elements to the heap using the heappush() function. This function takes two arguments: the heap and the element to be added. Here is an example:
import heapq
# create a heap
heap = []
# add elements to the heap
heapq.heappush(heap, 3)
heapq.heappush(heap, 1)
heapq.heappush(heap, 4)
# print the heap
print(heap)
This will output the following:
[1, 3, 4]
As you can see, the elements have been added to the heap in the correct order.
You can also remove the smallest element from the heap using the heappop() function. This function takes the heap as an argument and returns the smallest element. Here is an example:
import heapq
# create a heap
heap = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
# convert the list into a heap
heapq.heapify(heap)
# remove the smallest element from the heap
smallest = heapq.heappop(heap)
# print the smallest element
print(smallest)
# print the heap
print(heap)
This will output the following:
1
[1, 3, 2, 5, 5, 9, 4, 6, 5, 3]
As you can see, the smallest element has been removed from the heap, and the remaining elements have been reorganized to maintain the heap property.
What is a Heap in Python?
In conclusion, a Heap in Python is a data structure that allows for efficient access and manipulation of elements based on their priority. It is a binary tree that satisfies the heap property, which means that the parent node is always greater than or equal to its child nodes. Heaps are commonly used in algorithms such as Dijkstra’s shortest path algorithm and heap sort. Python provides a built-in module called “heapq” that allows for easy implementation of heaps. By understanding the concept of heaps and how to use them in Python, developers can improve the efficiency and performance of their code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |