A Heap is a specialized tree-based data structure that is used to efficiently manage and organize a collection of elements. It is a complete binary tree where each node has a value greater than or equal to its children (in a max heap) or less than or equal to its children (in a min heap). The root node of the heap always contains the highest (or lowest) value in the heap, making it useful for implementing priority queues and sorting algorithms. Heaps can be implemented using arrays or linked lists and have a time complexity of O(log n) for insertion, deletion, and search operations. Keep reading below to learn how to use a Heap in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
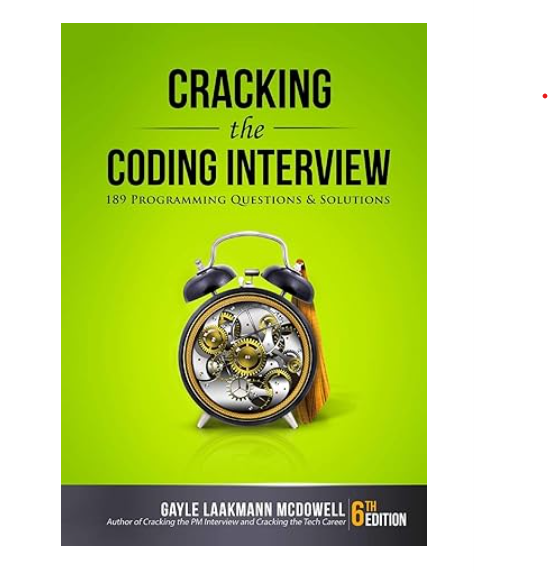
How to use a Heap in Rust with example code
A heap is a data structure that allows for efficient insertion and removal of elements in a specific order. In Rust, the standard library provides a binary heap implementation that can be used for this purpose.
To use a heap in Rust, you first need to import the `collections::BinaryHeap` module. Once you have done this, you can create a new heap by calling the `BinaryHeap::new()` function. By default, the heap will be a max heap, meaning that the largest element will be at the top.
Here is an example of how to create a new heap and insert some elements into it:
use std::collections::BinaryHeap;
let mut heap = BinaryHeap::new();
heap.push(5);
heap.push(2);
heap.push(10);
In this example, we create a new heap and insert the elements 5, 2, and 10 into it using the `push()` method. The heap will automatically reorder the elements so that the largest element (10) is at the top.
To remove elements from the heap, you can use the `pop()` method. This will remove the largest element from the heap and return it. Here is an example:
let largest = heap.pop().unwrap();
println!("The largest element is {}", largest);
In this example, we remove the largest element from the heap and print its value using the `println!()` macro.
Overall, using a heap in Rust is a simple and efficient way to manage a collection of elements in a specific order.
What is a Heap in Rust?
In conclusion, a heap in Rust is a data structure that allows for dynamic memory allocation. It is a region of memory that is managed by the operating system and can be accessed by the program at runtime. Rust provides a safe and efficient way to work with the heap through its ownership and borrowing system. By using Rust’s built-in memory management features, developers can avoid common issues such as memory leaks and buffer overflows. Overall, understanding how the heap works in Rust is essential for building reliable and performant applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |