A linked list is a linear data structure in computer science that consists of a sequence of nodes, where each node contains a data element and a reference (or pointer) to the next node in the sequence. Unlike arrays, linked lists do not have a fixed size and can be dynamically resized during runtime. Linked lists are commonly used in computer science for implementing various data structures such as stacks, queues, and hash tables. The main advantage of linked lists is their ability to efficiently insert and delete elements from the list, as well as their flexibility in terms of size and structure. Keep reading below to learn how to use a Linked List in Java.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
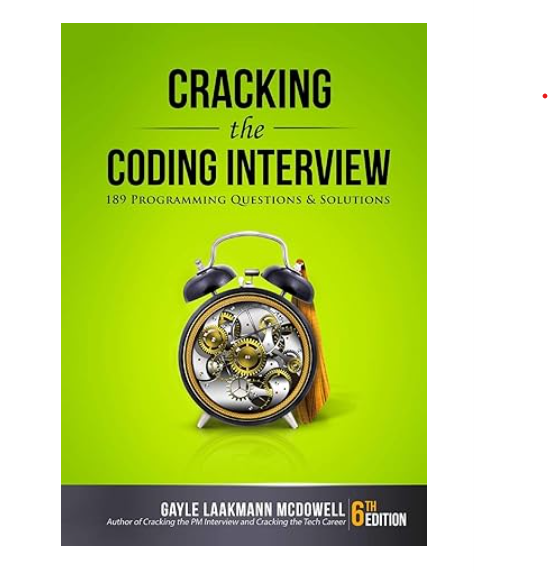
How to use a Linked List in Java with example code
A linked list is a data structure that consists of a sequence of nodes, where each node contains a reference to the next node in the list. In Java, a linked list can be implemented using the LinkedList class, which is part of the Java Collections Framework.
To use a linked list in Java, you first need to create an instance of the LinkedList class. You can then add elements to the list using the add() method, and remove elements using the remove() method. Here’s an example:
LinkedList
list.add("apple");
list.add("banana");
list.add("cherry");
list.remove("banana");
In this example, we create a new LinkedList object called “list”, and add three elements to it using the add() method. We then remove the element “banana” using the remove() method.
You can also iterate over the elements in a linked list using a for-each loop or an iterator. Here’s an example:
for (String fruit : list) {
System.out.println(fruit);
}
Iterator
while (iterator.hasNext()) {
String fruit = iterator.next();
System.out.println(fruit);
}
In this example, we use a for-each loop to iterate over the elements in the list and print them to the console. We also use an iterator to do the same thing.
Linked lists are useful when you need to add or remove elements from a list frequently, as they allow for efficient insertion and deletion operations. However, they can be slower than arrays when it comes to accessing elements at a specific index, as you need to traverse the list from the beginning to find the element you’re looking for.
What is a Linked List in Java?
In conclusion, a Linked List in Java is a data structure that allows for efficient insertion and deletion of elements. It consists of a series of nodes, each containing a value and a reference to the next node in the list. This structure is particularly useful when dealing with large amounts of data that need to be constantly updated or modified. While it may not be as efficient for accessing elements as an array, its flexibility and ease of use make it a valuable tool for any Java programmer. By understanding the basics of Linked Lists in Java, developers can create more efficient and effective programs that can handle complex data structures with ease.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |