A linked list is a linear data structure in computer science that consists of a sequence of nodes, where each node contains a data element and a reference (or pointer) to the next node in the sequence. Unlike arrays, linked lists do not have a fixed size and can be dynamically resized during runtime. Linked lists are commonly used in computer science for implementing various data structures such as stacks, queues, and hash tables. The main advantage of linked lists is their ability to efficiently insert and delete elements from the list, as well as their flexibility in terms of size and structure. Keep reading below to learn how to use a Linked List in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
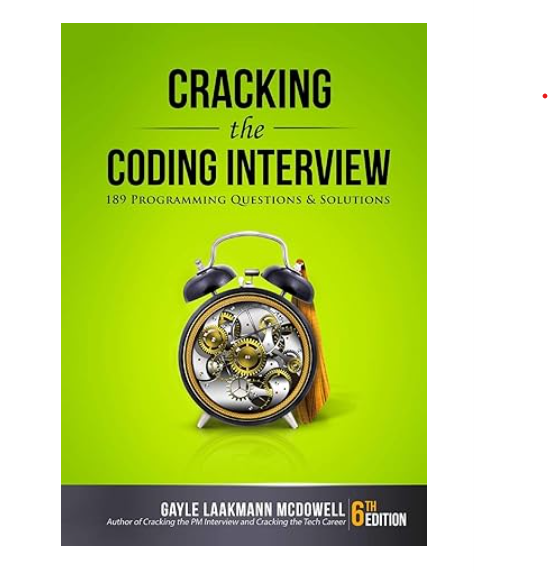
How to use a Linked List in Kotlin with example code
Linked lists are a fundamental data structure in computer science. They are used to store a collection of elements in a linear order. In this blog post, we will explore how to use a linked list in Kotlin.
To get started, we need to create a class for our linked list. We will call this class `LinkedList`. Our `LinkedList` class will have two properties: `head` and `tail`. The `head` property will point to the first node in the list, and the `tail` property will point to the last node in the list.
“`kotlin
class LinkedList
var head: Node
var tail: Node
}
“`
Next, we need to create a class for our nodes. We will call this class `Node`. Our `Node` class will have two properties: `value` and `next`. The `value` property will store the value of the node, and the `next` property will point to the next node in the list.
“`kotlin
class Node
var next: Node
}
“`
Now that we have our `LinkedList` and `Node` classes, we can start adding elements to our linked list. To add an element to the end of the list, we need to create a new node and set the `next` property of the current tail to the new node. We then set the `tail` property to the new node.
“`kotlin
fun add(value: T) {
val newNode = Node(value)
if (head == null) {
head = newNode
tail = newNode
} else {
tail?.next = newNode
tail = newNode
}
}
“`
To print the elements of our linked list, we can create a `toString()` method that iterates through the list and concatenates the values of each node.
“`kotlin
override fun toString(): String {
var current = head
var result = “”
while (current != null) {
result += “${current.value} ”
current = current.next
}
return result.trim()
}
“`
Let’s put it all together and create a simple example:
“`kotlin
fun main() {
val list = LinkedList
list.add(1)
list.add(2)
list.add(3)
println(list) // Output: 1 2 3
}
“`
In this example, we create a new `LinkedList` and add the values 1, 2, and 3 to the list. We then print the elements of the list using the `toString()` method we created earlier.
In conclusion, linked lists are a powerful data structure that can be used in a variety of applications. By creating a `LinkedList` class and a `Node` class, we can easily create and manipulate linked lists in Kotlin.
What is a Linked List in Kotlin?
In conclusion, a Linked List is a fundamental data structure in computer science that is used to store and manage collections of data. In Kotlin, a Linked List is implemented using a class that contains a reference to the first node in the list. Each node in the list contains a reference to the next node, allowing for efficient traversal and manipulation of the list. Linked Lists are particularly useful when dealing with large amounts of data that need to be dynamically added or removed from the list. By understanding the basics of Linked Lists in Kotlin, developers can create more efficient and effective code for their applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |