A linked list is a linear data structure in computer science that consists of a sequence of nodes, where each node contains a data element and a reference (or pointer) to the next node in the sequence. Unlike arrays, linked lists do not have a fixed size and can be dynamically resized during runtime. Linked lists are commonly used in computer science for implementing various data structures such as stacks, queues, and hash tables. The main advantage of linked lists is their ability to efficiently insert and delete elements from the list, as well as their flexibility in terms of size and structure. Keep reading below to learn how to use a Linked List in PHP.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
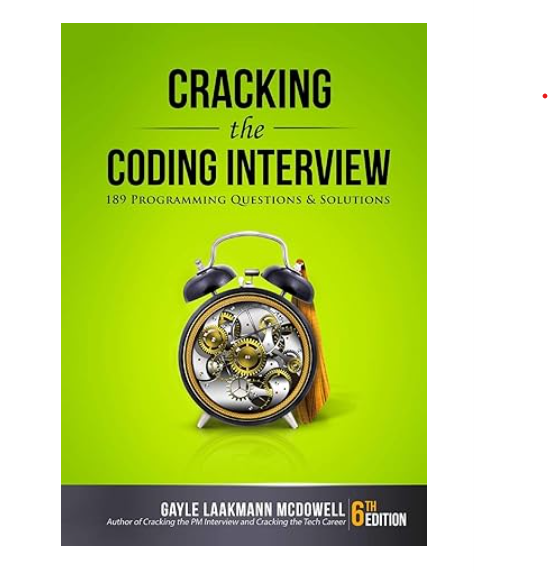
How to use a Linked List in PHP with example code
A linked list is a data structure that consists of a sequence of nodes, where each node contains a value and a reference to the next node in the sequence. In PHP, a linked list can be implemented using classes and objects.
To create a linked list in PHP, we first need to define a class for the nodes. The class should have two properties: a value property to store the value of the node, and a next property to store a reference to the next node in the sequence. Here’s an example:
class Node {
public $value;
public $next;
public function __construct($value) {
$this->value = $value;
$this->next = null;
}
}
Next, we need to define a class for the linked list itself. The class should have a head property to store a reference to the first node in the sequence, and methods to add nodes to the list and print the list. Here’s an example:
class LinkedList {
public $head;
public function __construct() {
$this->head = null;
}
public function add($value) {
$node = new Node($value);
if ($this->head === null) {
$this->head = $node;
} else {
$current = $this->head;
while ($current->next !== null) {
$current = $current->next;
}
$current->next = $node;
}
}
public function printList() {
$current = $this->head;
while ($current !== null) {
echo $current->value . " ";
$current = $current->next;
}
}
}
To use the linked list, we can create an instance of the LinkedList class and add some nodes to it:
$list = new LinkedList();
$list->add(1);
$list->add(2);
$list->add(3);
We can then print the list to see the values of the nodes:
$list->printList(); // Output: 1 2 3
Linked lists can be useful for a variety of applications, such as implementing stacks and queues, and for handling large amounts of data that may need to be dynamically resized.
What is a Linked List in PHP?
In conclusion, a linked list is a fundamental data structure in computer science that allows for efficient storage and manipulation of data. In PHP, a linked list can be implemented using classes and objects, with each node representing an object that contains a value and a reference to the next node in the list. Linked lists are particularly useful for scenarios where data needs to be inserted or removed frequently, as they allow for constant time complexity for these operations. By understanding the basics of linked lists in PHP, developers can create more efficient and scalable applications that can handle large amounts of data with ease.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |