A linked list is a linear data structure in computer science that consists of a sequence of nodes, where each node contains a data element and a reference (or pointer) to the next node in the sequence. Unlike arrays, linked lists do not have a fixed size and can be dynamically resized during runtime. Linked lists are commonly used in computer science for implementing various data structures such as stacks, queues, and hash tables. The main advantage of linked lists is their ability to efficiently insert and delete elements from the list, as well as their flexibility in terms of size and structure. Keep reading below to learn how to use a Linked List in Python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
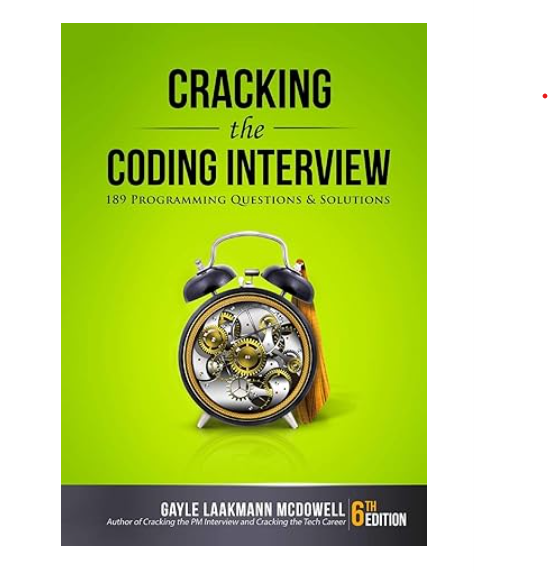
How to use a Linked List in Python with example code
A linked list is a data structure that consists of a sequence of nodes, where each node contains a value and a reference to the next node in the sequence. In Python, we can implement a linked list using classes.
To create a linked list, we first define a Node class that represents a single node in the list. The Node class has two attributes: a value and a next reference.
class Node:
def __init__(self, value):
self.value = value
self.next = None
Next, we define a LinkedList class that represents the entire list. The LinkedList class has a single attribute: a reference to the first node in the list.
class LinkedList:
def __init__(self):
self.head = None
To add a new node to the list, we create a new Node object and set its value. Then, we set the next reference of the new node to the current head of the list, and set the head of the list to the new node.
def add_node(self, value):
new_node = Node(value)
new_node.next = self.head
self.head = new_node
To print the contents of the list, we start at the head of the list and follow the next references until we reach the end of the list.
def print_list(self):
current = self.head
while current:
print(current.value)
current = current.next
Here’s an example of how to use the LinkedList class:
my_list = LinkedList()
my_list.add_node(3)
my_list.add_node(7)
my_list.add_node(1)
my_list.print_list()
This will output:
1
7
3
In this example, we created a new linked list called my_list and added three nodes to it with values 3, 7, and 1. We then printed the contents of the list, which were 1, 7, and 3 in that order.
What is a Linked List in Python?
In conclusion, a linked list is a fundamental data structure in computer science that allows for efficient storage and manipulation of data. In Python, a linked list can be implemented using classes and objects, with each node containing a value and a reference to the next node in the list. Linked lists are particularly useful when dealing with large amounts of data that need to be accessed and modified frequently, as they allow for constant time insertion and deletion operations. While they may not be as intuitive as other data structures like arrays, linked lists are an essential tool for any programmer looking to optimize their code and improve its performance.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |