A linked list is a linear data structure in computer science that consists of a sequence of nodes, where each node contains a data element and a reference (or pointer) to the next node in the sequence. Unlike arrays, linked lists do not have a fixed size and can be dynamically resized during runtime. Linked lists are commonly used in computer science for implementing various data structures such as stacks, queues, and hash tables. The main advantage of linked lists is their ability to efficiently insert and delete elements from the list, as well as their flexibility in terms of size and structure. Keep reading below to learn how to use a Linked List in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
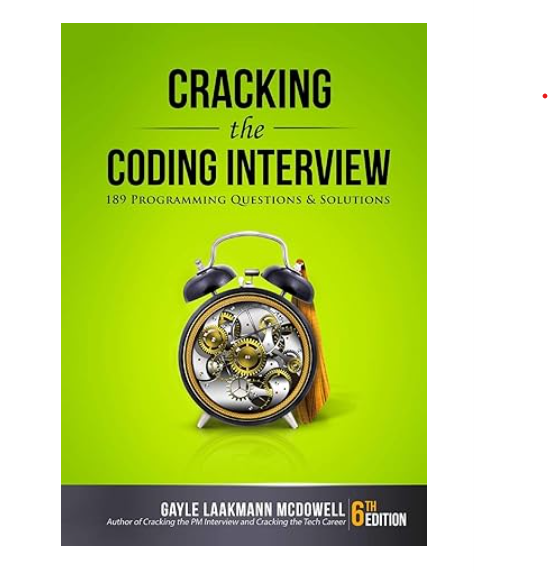
How to use a Linked List in Rust with example code
Linked lists are a fundamental data structure in computer science. They are used to store a collection of elements where each element points to the next one in the list. In Rust, linked lists can be implemented using the `std::collections::LinkedList` module.
To use the `LinkedList` module, you first need to import it into your Rust program:
use std::collections::LinkedList;
Once you have imported the module, you can create a new linked list using the `LinkedList::new()` method:
let mut list = LinkedList::new();
This creates a new, empty linked list. You can add elements to the list using the `push_back()` method:
list.push_back(1);
This adds the element `1` to the end of the list. You can add multiple elements at once using the `extend()` method:
list.extend([2, 3, 4].iter().cloned());
This adds the elements `2`, `3`, and `4` to the end of the list.
You can access elements in the list using the `get()` method, which returns an `Option<&T>`:
let second_element = list.get(1);
This retrieves the second element in the list (remember that Rust uses zero-based indexing). If the index is out of bounds, `get()` returns `None`.
You can also remove elements from the list using the `pop_front()` and `pop_back()` methods:
let first_element = list.pop_front();
This removes the first element from the list and returns it. If the list is empty, `pop_front()` returns `None`.
Overall, linked lists are a useful data structure to have in your Rust programming toolkit. They can be used to implement a variety of algorithms and data structures, and the `std::collections::LinkedList` module provides a convenient way to work with them.
What is a Linked List in Rust?
In conclusion, a linked list is a fundamental data structure in computer science that allows for efficient insertion and deletion of elements. In Rust, linked lists can be implemented using the standard library’s LinkedList module or by creating a custom implementation using Rust’s powerful memory management features. While linked lists may not be the most efficient data structure for all use cases, they are an important tool to have in your programming arsenal. By understanding the basics of linked lists in Rust, you can improve your ability to solve complex programming problems and build more efficient and effective software.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |