In computer science, a lock is a synchronization mechanism used to enforce mutual exclusion and prevent race conditions. It is a data structure that allows only one thread or process to access a shared resource at a time. When a thread or process acquires a lock, it gains exclusive access to the resource and all other threads or processes are blocked from accessing it until the lock is released. Locks can be implemented using various algorithms and data structures, such as semaphores, mutexes, and spin locks. They are commonly used in multi-threaded and distributed systems to ensure data consistency and prevent conflicts. Keep reading below to learn how to use a Lock in Bash.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
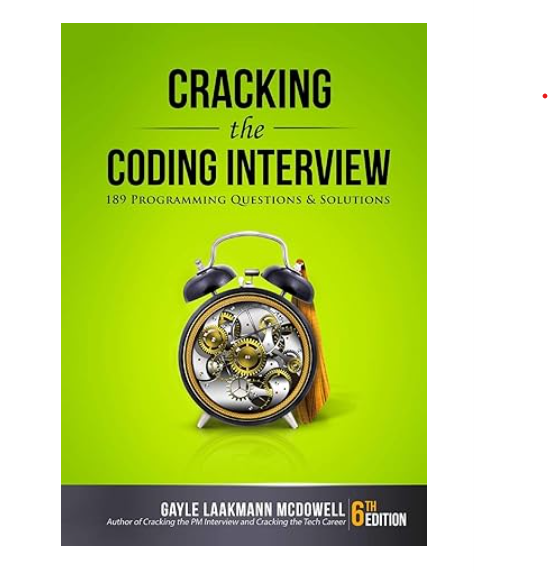
How to use a Lock in Bash with example code
A lock in Bash is a mechanism that allows only one instance of a script to run at a time. This can be useful in situations where multiple instances of a script could cause issues, such as when modifying a shared resource. In this blog post, we will discuss how to use a lock in Bash with example code.
To implement a lock in Bash, we can use a file as a lock. The script will create a file when it starts running, and delete the file when it finishes. If another instance of the script tries to run while the lock file exists, it will know that another instance is already running and exit.
Here is an example of how to implement a lock in Bash:
#!/bin/bash
LOCK_FILE=/tmp/my_script.lock
# Check if lock file exists
if [ -f $LOCK_FILE ]; then
echo "Script is already running."
exit 1
fi
# Create lock file
touch $LOCK_FILE
# Do some work here
# Remove lock file
rm $LOCK_FILE
In this example, we first define the path to the lock file. We then check if the lock file exists. If it does, we print an error message and exit. If it doesn’t, we create the lock file using the touch command.
We then do some work in the script, and finally remove the lock file using the rm command.
By using a lock in Bash, we can ensure that only one instance of a script is running at a time, which can help prevent issues caused by multiple instances modifying a shared resource.
What is a Lock in Bash?
In conclusion, a lock in Bash is a mechanism that allows you to prevent multiple instances of a script or program from running simultaneously. It is a useful tool for ensuring that critical processes are not interrupted or corrupted by multiple instances running at the same time. By using locks, you can ensure that only one instance of a script or program is running at any given time, which can help to prevent errors and improve the overall stability of your system. Whether you are a seasoned Bash user or just getting started, understanding how to use locks can be an important skill to have in your toolkit. So, if you want to ensure that your scripts and programs run smoothly and without interruption, be sure to learn how to use locks in Bash.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |