In computer science, a lock is a synchronization mechanism used to enforce mutual exclusion and prevent race conditions. It is a data structure that allows only one thread or process to access a shared resource at a time. When a thread or process acquires a lock, it gains exclusive access to the resource and all other threads or processes are blocked from accessing it until the lock is released. Locks can be implemented using various algorithms and data structures, such as semaphores, mutexes, and spin locks. They are commonly used in multi-threaded and distributed systems to ensure data consistency and prevent conflicts. Keep reading below to learn how to use a Lock in Java.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
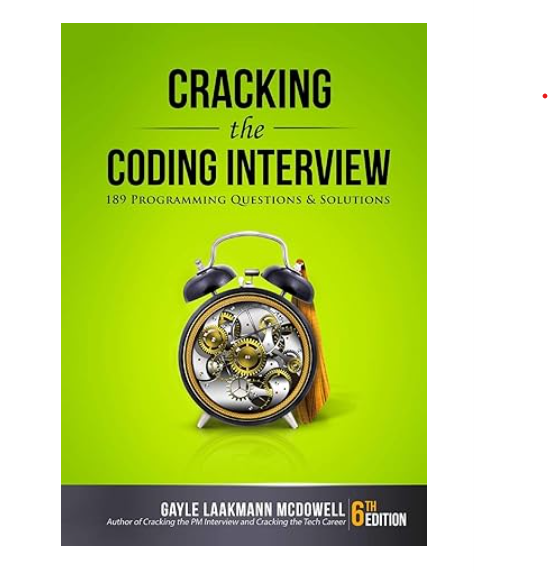
How to use a Lock in Java with example code
Java provides a Lock interface that can be used to synchronize access to a shared resource. The Lock interface provides more functionality than the synchronized keyword, such as the ability to interrupt a thread waiting for a lock and the ability to try to acquire a lock without blocking. In this blog post, we will discuss how to use a Lock in Java with example code.
To use a Lock, you first need to create an instance of a class that implements the Lock interface. The most commonly used implementation is the ReentrantLock class. Here is an example of how to create a ReentrantLock:
Lock lock = new ReentrantLock();
To acquire a lock, you call the lock() method on the Lock object. This method will block until the lock is available. Here is an example:
lock.lock();
Once you have acquired the lock, you can access the shared resource. When you are done accessing the shared resource, you should release the lock by calling the unlock() method. Here is an example:
lock.unlock();
It is important to always release the lock, even if an exception is thrown while accessing the shared resource. To ensure that the lock is always released, you can use a try-finally block:
lock.lock();
try {
// access the shared resource
} finally {
lock.unlock();
}
In addition to the lock() and unlock() methods, the Lock interface provides other methods that can be useful in certain situations. For example, the tryLock() method can be used to try to acquire a lock without blocking. This method returns true if the lock was acquired and false otherwise. Here is an example:
if (lock.tryLock()) {
try {
// access the shared resource
} finally {
lock.unlock();
}
} else {
// do something else
}
In conclusion, the Lock interface provides a powerful mechanism for synchronizing access to a shared resource in Java. By using a Lock, you can avoid some of the limitations of the synchronized keyword and have more control over the synchronization process.
What is a Lock in Java?
In conclusion, a lock in Java is a synchronization mechanism that allows multiple threads to access a shared resource in a mutually exclusive manner. It ensures that only one thread can access the resource at a time, preventing race conditions and other concurrency issues. Java provides several types of locks, including intrinsic locks, explicit locks, and read-write locks, each with its own advantages and disadvantages. Understanding how locks work and when to use them is essential for writing efficient and thread-safe Java code. By using locks effectively, developers can ensure that their applications are scalable, reliable, and performant in multi-threaded environments.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |