In computer science, a lock is a synchronization mechanism used to enforce mutual exclusion and prevent race conditions. It is a data structure that allows only one thread or process to access a shared resource at a time. When a thread or process acquires a lock, it gains exclusive access to the resource and all other threads or processes are blocked from accessing it until the lock is released. Locks can be implemented using various algorithms and data structures, such as semaphores, mutexes, and spin locks. They are commonly used in multi-threaded and distributed systems to ensure data consistency and prevent conflicts. Keep reading below to learn how to use a Lock in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
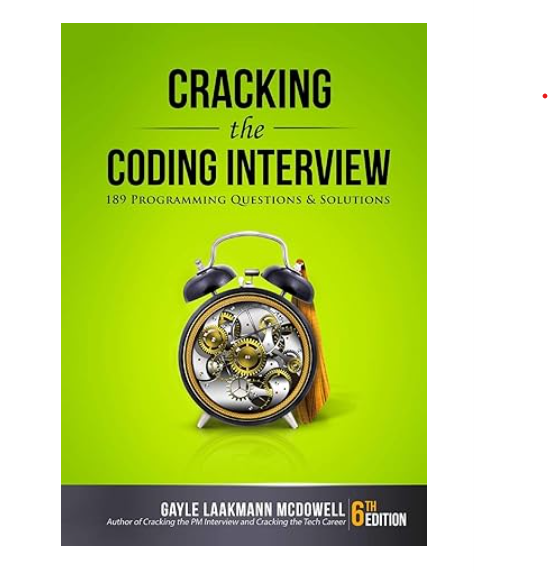
How to use a Lock in Kotlin with example code
Kotlin is a modern programming language that is gaining popularity among developers. One of the features that Kotlin provides is the ability to use locks to synchronize access to shared resources. In this blog post, we will discuss how to use locks in Kotlin with an example code.
Locks are used to synchronize access to shared resources in a multi-threaded environment. In Kotlin, locks can be implemented using the `synchronized` keyword. The `synchronized` keyword is used to create a block of code that can only be executed by one thread at a time.
Here is an example code that demonstrates the use of locks in Kotlin:
class Counter {
private var count = 0
fun increment() {
synchronized(this) {
count++
}
}
fun getCount(): Int {
synchronized(this) {
return count
}
}
}
fun main() {
val counter = Counter()
// Create 10 threads that increment the counter
val threads = List(10) {
Thread {
for (i in 1..1000) {
counter.increment()
}
}
}
// Start the threads
threads.forEach { it.start() }
// Wait for the threads to finish
threads.forEach { it.join() }
// Print the final count
println("Final count: ${counter.getCount()}")
}
In this example, we have a `Counter` class that has two methods: `increment()` and `getCount()`. The `increment()` method increments the `count` variable by one, and the `getCount()` method returns the current value of the `count` variable.
To ensure that the `increment()` and `getCount()` methods are executed by only one thread at a time, we have used the `synchronized` keyword with the `this` keyword as the lock object. This ensures that only one thread can execute the block of code inside the `synchronized` block at a time.
In the `main()` function, we create 10 threads that increment the counter 1000 times each. We then start the threads and wait for them to finish using the `join()` method. Finally, we print the final value of the counter using the `getCount()` method.
In conclusion, locks are an important feature in multi-threaded programming, and Kotlin provides an easy way to use locks using the `synchronized` keyword. By using locks, we can ensure that shared resources are accessed in a thread-safe manner.
What is a Lock in Kotlin?
In conclusion, a lock in Kotlin is a synchronization mechanism that allows multiple threads to access a shared resource in a mutually exclusive manner. It ensures that only one thread can access the resource at a time, preventing race conditions and other concurrency issues. Kotlin provides several types of locks, including the synchronized keyword, the ReentrantLock class, and the ReadWriteLock interface. Each of these locks has its own advantages and disadvantages, and the choice of which one to use depends on the specific requirements of the application. By using locks effectively, developers can write concurrent code that is both efficient and safe.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |