In computer science, a lock is a synchronization mechanism used to enforce mutual exclusion and prevent race conditions. It is a data structure that allows only one thread or process to access a shared resource at a time. When a thread or process acquires a lock, it gains exclusive access to the resource and all other threads or processes are blocked from accessing it until the lock is released. Locks can be implemented using various algorithms and data structures, such as semaphores, mutexes, and spin locks. They are commonly used in multi-threaded and distributed systems to ensure data consistency and prevent conflicts. Keep reading below to learn how to use a Lock in PHP.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
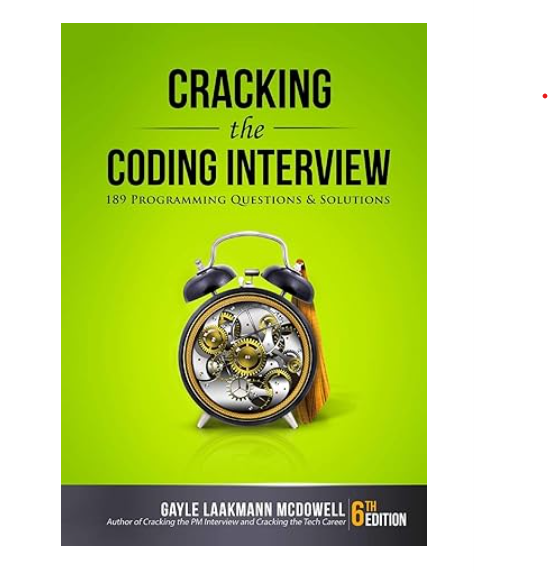
How to use a Lock in PHP with example code
A lock is a synchronization mechanism that is used to prevent multiple threads from accessing shared resources simultaneously. In PHP, locks can be implemented using the `flock()` function.
To use a lock in PHP, you first need to open the file that you want to lock using the `fopen()` function. Once the file is open, you can use the `flock()` function to acquire a lock on the file.
Here’s an example code snippet that demonstrates how to use a lock in PHP:
$file = fopen("example.txt", "r+");
if (flock($file, LOCK_EX)) {
// Do some critical section work here
flock($file, LOCK_UN); // Release the lock
} else {
echo "Could not acquire lock!";
}
fclose($file);
In this example, we open the file “example.txt” in read/write mode using the `fopen()` function. We then use the `flock()` function with the `LOCK_EX` flag to acquire an exclusive lock on the file. This means that no other process can access the file until we release the lock.
Once we have acquired the lock, we can perform some critical section work on the file. Once we are done, we release the lock using the `flock()` function with the `LOCK_UN` flag.
It’s important to note that locks are only effective if all processes that access the shared resource use them. If one process accesses the resource without acquiring a lock, it can cause race conditions and other synchronization issues.
What is a Lock in PHP?
In conclusion, a lock in PHP is a mechanism that allows for the synchronization of concurrent processes or threads. It ensures that only one process or thread can access a shared resource at a time, preventing conflicts and data corruption. Locks can be implemented using various techniques such as file locking, database locking, and semaphore locking. It is important to use locks in PHP applications that involve multiple processes or threads to ensure data consistency and prevent race conditions. By understanding the concept of locks and implementing them correctly, developers can create robust and reliable PHP applications that can handle high levels of concurrency.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |