In computer science, a lock is a synchronization mechanism used to enforce mutual exclusion and prevent race conditions. It is a data structure that allows only one thread or process to access a shared resource at a time. When a thread or process acquires a lock, it gains exclusive access to the resource and all other threads or processes are blocked from accessing it until the lock is released. Locks can be implemented using various algorithms and data structures, such as semaphores, mutexes, and spin locks. They are commonly used in multi-threaded and distributed systems to ensure data consistency and prevent conflicts. Keep reading below to learn how to use a Lock in Python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
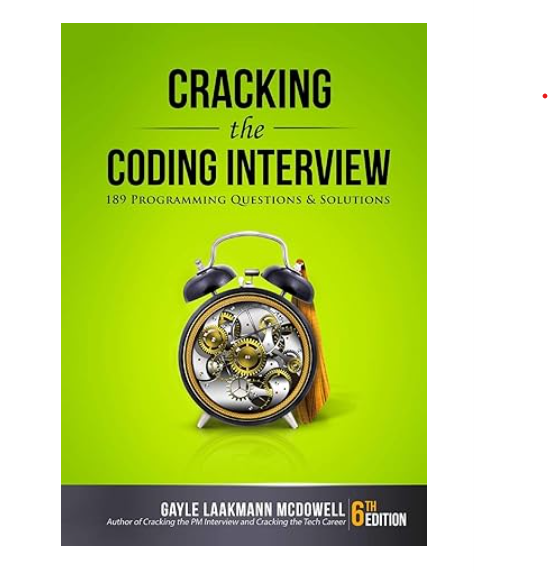
How to use a Lock in Python with example code
Python is a versatile programming language that can be used for a variety of tasks. One common task is locking resources to prevent multiple processes from accessing them simultaneously. In this blog post, we will discuss how to use a lock in Python.
A lock is a synchronization primitive that is used to prevent multiple processes from accessing a shared resource simultaneously. In Python, locks are implemented using the `threading` module. The `threading` module provides a `Lock` class that can be used to create locks.
To use a lock in Python, you first need to create an instance of the `Lock` class. You can do this using the following code:
lock = threading.Lock()
Once you have created a lock, you can use it to synchronize access to a shared resource. To acquire the lock, you can use the `acquire()` method of the lock object. This method will block until the lock is available. Once the lock is acquired, you can access the shared resource. After you are done accessing the shared resource, you should release the lock using the `release()` method. Here is an example:
import threading
lock = threading.Lock()
def my_function():
lock.acquire()
# Access shared resource here
lock.release()
In this example, the `my_function()` function acquires the lock before accessing the shared resource and releases the lock after it is done.
It is important to note that locks can cause deadlocks if they are not used correctly. A deadlock occurs when two or more processes are waiting for each other to release a lock. To avoid deadlocks, you should always acquire locks in a consistent order and release them in the reverse order.
In conclusion, locks are an important tool for synchronizing access to shared resources in Python. By using the `Lock` class from the `threading` module, you can prevent multiple processes from accessing a shared resource simultaneously. Just remember to use locks correctly to avoid deadlocks.
What is a Lock in Python?
In conclusion, a lock in Python is a synchronization mechanism that helps to prevent multiple threads from accessing shared resources simultaneously. It ensures that only one thread can access the shared resource at a time, thereby preventing race conditions and other concurrency issues. Locks are an essential tool for building robust and reliable multi-threaded applications in Python. By using locks, developers can ensure that their code is thread-safe and can handle multiple concurrent requests without any issues. Overall, understanding how locks work and how to use them effectively is crucial for any Python developer who wants to build high-performance and scalable applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |