A Map is a data structure in computer science that stores key-value pairs. It allows for efficient lookup, insertion, and deletion of elements based on their keys. Maps are commonly used in programming to represent relationships between different entities, such as a dictionary of words and their definitions or a database of customer information. Maps can be implemented using various data structures, such as hash tables or binary search trees, and are an essential tool for solving many computational problems. Keep reading below to learn how to use a Map in Bash.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
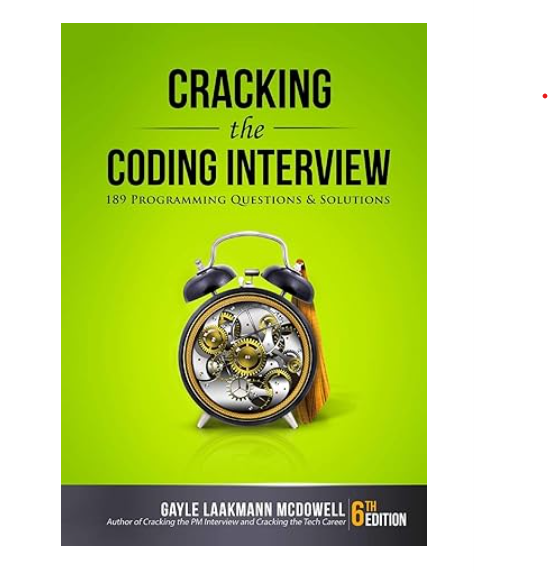
How to use a Map in Bash with example code
Bash is a powerful scripting language that can be used to automate various tasks. One of the useful features of Bash is the ability to use maps. A map is a collection of key-value pairs that can be used to store and retrieve data efficiently. In this blog post, we will explore how to use maps in Bash with example code.
To create a map in Bash, we can use the `declare` command with the `-A` option. Here is an example:
declare -A my_map
This creates an empty map called `my_map`. We can add key-value pairs to the map using the following syntax:
my_map[key]=value
For example, let’s add some key-value pairs to `my_map`:
my_map["name"]="John"
my_map["age"]=30
We can retrieve the value of a key using the following syntax:
echo ${my_map[key]}
For example, to retrieve the value of the “name” key, we can use:
echo ${my_map["name"]}
This will output “John”.
We can also loop through all the keys in a map using the following syntax:
for key in "${!my_map[@]}"
do
echo "Key: $key Value: ${my_map[$key]}"
done
This will output:
Key: name Value: John
Key: age Value: 30
Maps can be very useful in Bash scripts, especially when dealing with large amounts of data. By using maps, we can store and retrieve data efficiently, making our scripts faster and more reliable.
In conclusion, we have learned how to use maps in Bash with example code. We can create maps using the `declare` command, add key-value pairs to the map, retrieve the value of a key, and loop through all the keys in a map. By using maps, we can make our Bash scripts more powerful and efficient.
What is a Map in Bash?
In conclusion, a map in Bash is a data structure that allows you to store and retrieve key-value pairs. It is a powerful tool that can simplify your Bash scripts and make them more efficient. With a map, you can easily access and manipulate data without having to write complex code. Whether you are working on a small project or a large-scale application, a map can be a valuable asset in your Bash toolkit. So, if you haven’t already, give maps a try in your next Bash script and see how they can improve your workflow.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |