A Map is a data structure in computer science that stores key-value pairs. It allows for efficient lookup, insertion, and deletion of elements based on their keys. Maps are commonly used in algorithms and data processing applications where fast access to data is required. They can be implemented using various data structures such as hash tables, binary search trees, or balanced trees. Maps are useful for a wide range of applications, including database indexing, caching, and data analysis. Keep reading below to learn how to use a Map in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
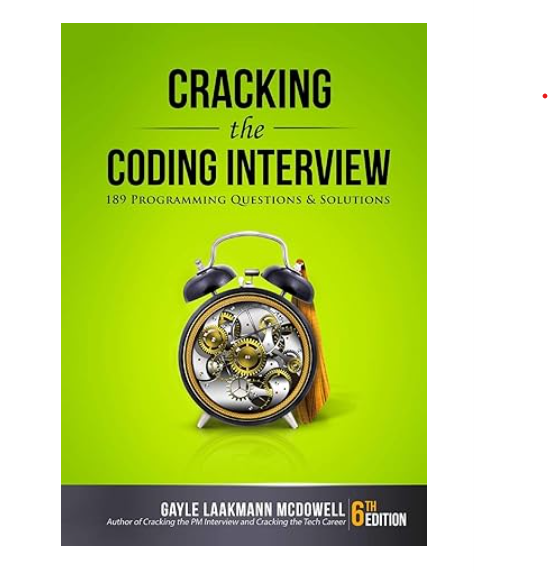
How to use a Map in C# with example code
Maps are a useful data structure in programming, allowing you to store and access key-value pairs. In C#, maps are implemented using the `Dictionary` class. Here’s how you can use a map in C#:
First, you need to create a new `Dictionary` object. You can specify the types of the keys and values when you create the dictionary, like this:
Dictionary<string, int> myDictionary = new Dictionary<string, int>();
This creates a new dictionary that maps strings to integers.
To add a new key-value pair to the dictionary, you can use the `Add` method:
myDictionary.Add("apple", 3);
This adds the key “apple” with the value 3 to the dictionary.
To access a value in the dictionary, you can use the square bracket notation:
int value = myDictionary["apple"];
This sets the variable `value` to 3, the value associated with the key “apple”.
You can also check if a key exists in the dictionary using the `ContainsKey` method:
if (myDictionary.ContainsKey("apple")) { ... }
This returns `true` if the key “apple” exists in the dictionary.
Finally, you can iterate over all the key-value pairs in the dictionary using a `foreach` loop:
foreach (KeyValuePair<string, int> pair in myDictionary) { ... }
Inside the loop, you can access the key and value using the `Key` and `Value` properties of the `KeyValuePair` object.
That’s a basic overview of how to use a map in C#. With this knowledge, you can start using dictionaries to store and access data in your own programs.
What is a Map in C#?
In conclusion, a map in C# is a powerful data structure that allows developers to store and manipulate key-value pairs. It provides a flexible and efficient way to organize and access data, making it an essential tool for many programming tasks. Whether you’re building a complex application or simply need to store some data, a map in C# can help you get the job done quickly and easily. With its intuitive syntax and robust functionality, it’s no wonder that maps are a popular choice among C# developers. So if you’re looking to take your programming skills to the next level, be sure to explore the many benefits of using a map in C#.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |