A Map is a data structure in computer science that stores key-value pairs. It allows for efficient lookup, insertion, and deletion of elements based on their keys. Maps are commonly used in programming to represent relationships between different entities, such as a dictionary of words and their definitions or a database of customer information. Maps can be implemented using various data structures, such as hash tables or binary search trees, and are an essential tool for solving many computational problems. Keep reading below to learn how to use a Map in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
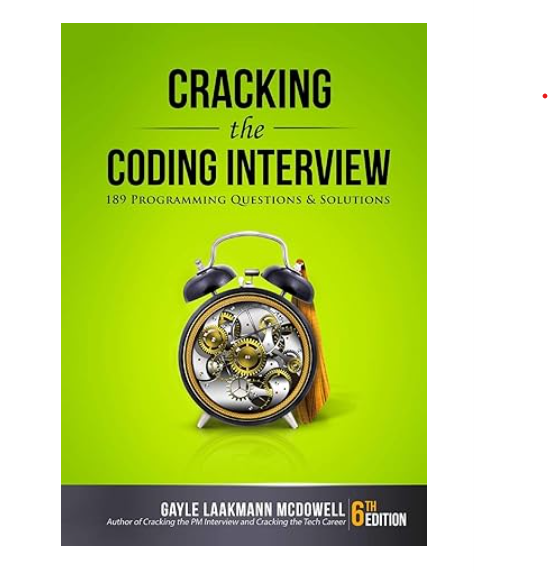
How to use a Map in TypeScript with example code
Maps are a useful data structure in TypeScript that allow you to store key-value pairs. They are similar to objects, but with a few key differences. In this blog post, we will explore how to use a Map in TypeScript with example code.
To create a new Map in TypeScript, you can use the `Map` constructor. Here is an example:
const myMap = new Map();
This creates a new empty Map. You can also initialize a Map with key-value pairs like this:
const myMap = new Map([
['key1', 'value1'],
['key2', 'value2']
]);
To add a new key-value pair to a Map, you can use the `set` method. Here is an example:
myMap.set('key3', 'value3');
To get the value associated with a key in a Map, you can use the `get` method. Here is an example:
const value = myMap.get('key1');
console.log(value); // Output: 'value1'
You can also check if a key exists in a Map using the `has` method. Here is an example:
const hasKey = myMap.has('key1');
console.log(hasKey); // Output: true
To delete a key-value pair from a Map, you can use the `delete` method. Here is an example:
myMap.delete('key1');
Finally, you can get the number of key-value pairs in a Map using the `size` property. Here is an example:
const size = myMap.size;
console.log(size); // Output: 2
In conclusion, Maps are a powerful data structure in TypeScript that allow you to store key-value pairs. They are easy to use and provide a lot of flexibility. We hope this blog post has been helpful in showing you how to use a Map in TypeScript.
What is a Map in TypeScript?
In conclusion, a map in TypeScript is a powerful data structure that allows developers to store and manipulate key-value pairs. It provides a flexible and efficient way to manage data, making it an essential tool for any TypeScript project. With its ability to handle complex data structures and its built-in methods for adding, removing, and updating elements, maps are a valuable addition to any developer’s toolkit. Whether you’re working on a small project or a large-scale application, understanding how to use maps in TypeScript can help you write more efficient and effective code. So, if you’re looking to improve your TypeScript skills, be sure to explore the many benefits of using maps in your next project.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |