A queue is a linear data structure in computer science that follows the First-In-First-Out (FIFO) principle. It is similar to a line of people waiting for a service, where the first person who joins the line is the first one to be served. In a queue, elements are added at the rear end and removed from the front end. The operations performed on a queue are enqueue, which adds an element to the rear end, and dequeue, which removes an element from the front end. Queues are commonly used in computer science for tasks such as job scheduling, network packet handling, and implementing algorithms like breadth-first search. Keep reading below to learn how to use a Queue in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
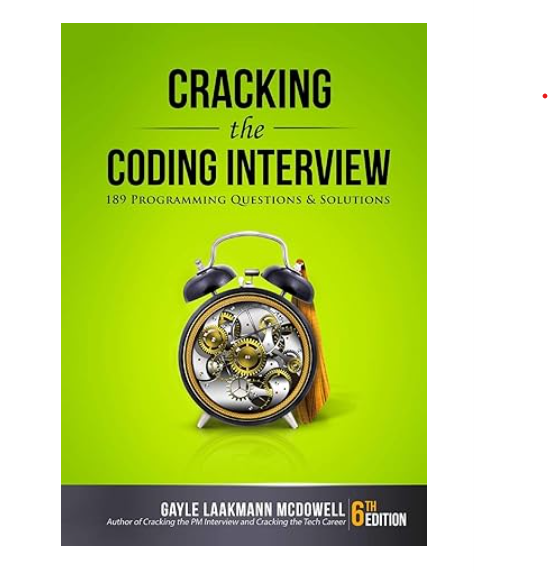
How to use a Queue in C# with example code
Queues are a fundamental data structure in computer science that allow for efficient processing of data in a first-in, first-out (FIFO) manner. In C#, the Queue class is available in the System.Collections namespace and provides a simple and efficient way to implement a queue.
To use a Queue in C#, you first need to create an instance of the Queue class. You can do this using the following code:
Queue<string> myQueue = new Queue<string>();
This creates a new Queue object that can hold strings. You can replace “string” with any other data type that you want to store in the queue.
Once you have created a Queue object, you can add items to the queue using the Enqueue method. For example:
myQueue.Enqueue("apple");
This adds the string “apple” to the end of the queue.
To remove an item from the queue, you can use the Dequeue method. This removes the first item in the queue and returns it. For example:
string firstItem = myQueue.Dequeue();
This removes the first item in the queue and stores it in the variable firstItem.
You can also check the number of items in the queue using the Count property. For example:
int numItems = myQueue.Count;
This stores the number of items in the queue in the variable numItems.
Overall, the Queue class in C# provides a simple and efficient way to implement a queue data structure. By using the Enqueue and Dequeue methods, you can easily add and remove items from the queue in a FIFO manner.
What is a Queue in C#?
In conclusion, a queue in C# is a data structure that allows for the efficient storage and retrieval of elements in a first-in, first-out (FIFO) manner. It is a useful tool for managing tasks or processes that need to be executed in a specific order. With its simple and intuitive implementation, a queue can be easily integrated into any C# program. Whether you are working on a small project or a large-scale application, understanding the basics of a queue can help you optimize your code and improve its performance. So, if you are looking for a reliable and efficient way to manage your data, consider using a queue in your C# programming.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |