A queue is a linear data structure in computer science that follows the First-In-First-Out (FIFO) principle. It is similar to a line of people waiting for a service, where the first person who joins the line is the first one to be served. In a queue, elements are added at the rear end and removed from the front end. The operations performed on a queue are enqueue, which adds an element to the rear end, and dequeue, which removes an element from the front end. Queues are commonly used in computer science for tasks such as job scheduling, network packet handling, and implementing algorithms like breadth-first search. Keep reading below to learn how to use a Queue in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
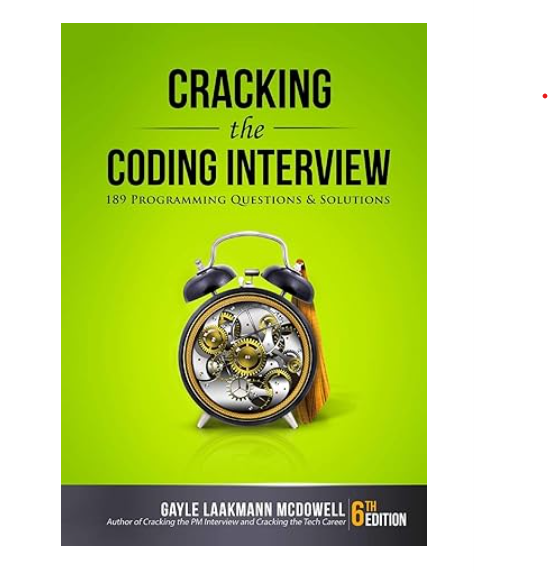
How to use a Queue in C++ with example code
Queues are a fundamental data structure in computer science that allow for efficient storage and retrieval of data. In C++, queues can be implemented using the standard library’s `queue` container. In this blog post, we will explore how to use a queue in C++ and provide example code to demonstrate its usage.
To use a queue in C++, we first need to include the `
#include
#include
int main() {
std::queue
return 0;
}
In this example, we create an empty queue of integers called `myQueue`. We can add elements to the queue using the `push()` method and remove elements from the queue using the `pop()` method. We can also access the front element of the queue using the `front()` method and check if the queue is empty using the `empty()` method.
Here is an example of how to add elements to a queue:
#include
#include
int main() {
std::queue
myQueue.push(1);
myQueue.push(2);
myQueue.push(3);
return 0;
}
In this example, we add the integers 1, 2, and 3 to the queue using the `push()` method. The elements are added to the back of the queue in the order they are pushed.
Here is an example of how to remove elements from a queue:
#include
#include
int main() {
std::queue
myQueue.push(1);
myQueue.push(2);
myQueue.push(3);
myQueue.pop();
return 0;
}
In this example, we remove the front element of the queue using the `pop()` method. The front element, which is the integer 1, is removed from the queue.
Here is an example of how to access the front element of a queue:
#include
#include
int main() {
std::queue
myQueue.push(1);
myQueue.push(2);
myQueue.push(3);
std::cout << myQueue.front() << std::endl;
return 0;
}
In this example, we access the front element of the queue using the `front()` method and print it to the console. The front element, which is the integer 1, is printed to the console.
Here is an example of how to check if a queue is empty:
#include
#include
int main() {
std::queue
std::cout << myQueue.empty() << std::endl;
return 0;
}
In this example, we check if the queue is empty using the `empty()` method and print the result to the console. Since the queue is empty, the result printed to the console is 1.
In conclusion, queues are a useful data structure in C++ that allow for efficient storage and retrieval of data. By using the `queue` container class provided by the standard library, we can easily create and manipulate queues in our C++ programs.
What is a Queue in C++?
In conclusion, a queue in C++ is a data structure that follows the First-In-First-Out (FIFO) principle. It is a container that allows elements to be inserted at the back and removed from the front. Queues are commonly used in programming for tasks such as managing resources, scheduling tasks, and implementing algorithms. C++ provides a built-in queue container that makes it easy to implement and use queues in your programs. By understanding the basics of queues and how they work in C++, you can improve the efficiency and functionality of your code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |