A queue is a linear data structure in computer science that follows the First-In-First-Out (FIFO) principle. It is similar to a line of people waiting for a service, where the first person who joins the line is the first one to be served. In a queue, elements are added at the rear end and removed from the front end. The operations performed on a queue are enqueue, which adds an element to the rear end, and dequeue, which removes an element from the front end. Queues are commonly used in computer science for tasks such as job scheduling, network packet handling, and implementing algorithms like breadth-first search. Keep reading below to learn how to use a Queue in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
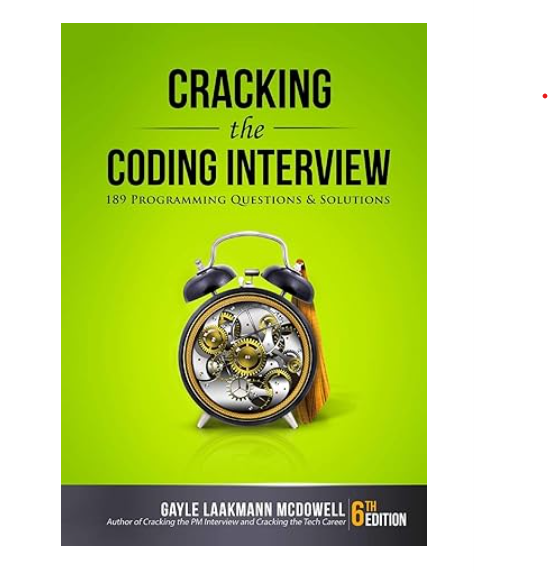
How to use a Queue in Go with example code
Queues are a fundamental data structure in computer science that allow for efficient processing of data in a first-in, first-out (FIFO) manner. In Go, queues can be implemented using slices or linked lists. In this blog post, we will explore how to use a queue in Go using a slice implementation.
To implement a queue using a slice, we can define a struct that contains a slice and two integers to keep track of the front and rear of the queue. Here’s an example:
type Queue struct {
items []int
front int
rear int
}
To enqueue an item, we can simply append it to the end of the slice and increment the rear index. Here’s the code:
func (q *Queue) Enqueue(item int) {
q.items = append(q.items, item)
q.rear++
}
To dequeue an item, we can retrieve the item at the front of the queue, increment the front index, and return the item. Here’s the code:
func (q *Queue) Dequeue() int {
if q.front == q.rear {
return -1 // queue is empty
}
item := q.items[q.front]
q.front++
return item
}
We can also implement other common queue operations such as checking if the queue is empty and getting the size of the queue. Here’s the code:
func (q *Queue) IsEmpty() bool {
return q.front == q.rear
}
func (q *Queue) Size() int {
return q.rear - q.front
}
Using a queue can be useful in many scenarios such as processing tasks in a background worker or implementing a breadth-first search algorithm. With this implementation, we can easily use a queue in our Go programs.
What is a Queue in Go?
In conclusion, a queue is a fundamental data structure that is widely used in computer science and programming. In Go, a queue can be implemented using various techniques such as slices, linked lists, or channels. Queues are useful for managing tasks that need to be executed in a specific order, and they can also be used for handling asynchronous events. By understanding the basics of queues in Go, developers can write more efficient and scalable code that can handle complex tasks with ease. Whether you are a beginner or an experienced programmer, mastering the concept of queues in Go is essential for building robust and reliable software applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |