A queue is a linear data structure in computer science that follows the First-In-First-Out (FIFO) principle. It is similar to a line of people waiting for a service, where the first person who joins the line is the first one to be served. In a queue, elements are added at the rear end and removed from the front end. The operations performed on a queue are enqueue, which adds an element to the rear end, and dequeue, which removes an element from the front end. Queues are commonly used in computer science for tasks such as job scheduling, network packet handling, and implementing algorithms like Breadth-First Search. Keep reading below to learn how to use a Queue in Java.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
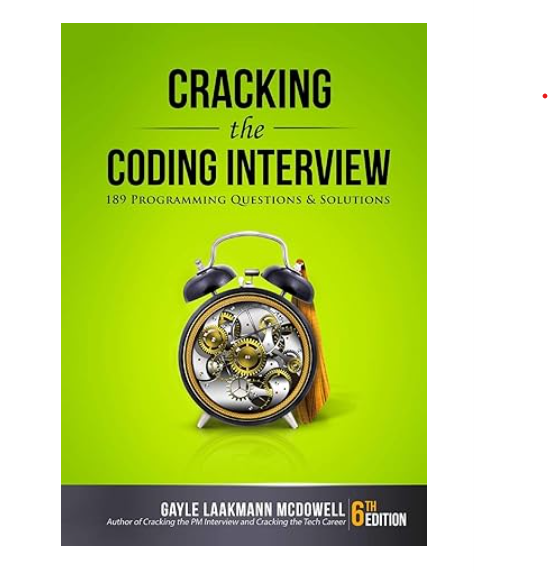
How to use a Queue in Java with example code
Queues are a fundamental data structure in computer science that allow for efficient processing of data in a first-in, first-out (FIFO) manner. In Java, the Queue interface is part of the java.util package and provides a set of methods for working with queues.
To use a Queue in Java, you first need to create an instance of a class that implements the Queue interface. One common implementation is the LinkedList class, which provides a doubly-linked list implementation of the Queue interface.
Here’s an example of how to create a Queue using the LinkedList class:
Queue<String> queue = new LinkedList<>();
This creates a new Queue that can hold elements of type String.
To add elements to the Queue, you can use the add() method:
queue.add("apple");
queue.add("banana");
queue.add("cherry");
This adds three elements to the Queue: “apple”, “banana”, and “cherry”.
To remove elements from the Queue, you can use the remove() method:
String first = queue.remove();
This removes the first element from the Queue and returns it. In this case, the value of first would be “apple”.
You can also use the peek() method to get the first element in the Queue without removing it:
String first = queue.peek();
This returns the first element in the Queue, but does not remove it. In this case, the value of first would be “banana”.
Overall, using a Queue in Java is a simple and effective way to manage data in a FIFO manner.
What is a Queue in Java?
In conclusion, a Queue in Java is a data structure that allows elements to be added and removed in a specific order. It follows the First-In-First-Out (FIFO) principle, meaning that the first element added to the queue will be the first one to be removed. Queues are commonly used in programming for tasks such as managing tasks in a system, processing messages, and implementing algorithms. Java provides a built-in Queue interface and several implementations, including LinkedList and PriorityQueue. Understanding how to use and implement Queues in Java can greatly improve the efficiency and functionality of your programs.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |