A queue is a linear data structure in computer science that follows the First-In-First-Out (FIFO) principle. It is similar to a line of people waiting for a service, where the first person who joins the line is the first one to be served. In a queue, elements are added at the rear end and removed from the front end. The operations performed on a queue are enqueue, which adds an element to the rear end, and dequeue, which removes an element from the front end. Queues are commonly used in computer science for tasks such as job scheduling, network packet handling, and implementing algorithms like breadth-first search. Keep reading below to learn how to use a Queue in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
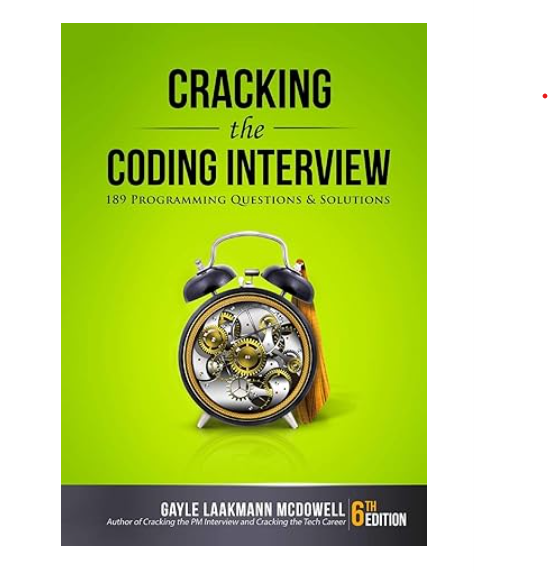
How to use a Queue in Rust with example code
Queues are a fundamental data structure in computer science that are used to store and manage collections of items. In Rust, queues can be implemented using the standard library’s `VecDeque` type.
To use a queue in Rust, you first need to import the `collections` module from the standard library:
use std::collections::VecDeque;
Once you have imported the `VecDeque` type, you can create a new queue by calling its `new` method:
let mut queue = VecDeque::new();
This creates a new, empty queue that can hold elements of any type. To add elements to the queue, you can use the `push_back` method:
queue.push_back(1);
This adds the integer `1` to the back of the queue. To remove elements from the queue, you can use the `pop_front` method:
let first_element = queue.pop_front();
This removes the first element from the queue and returns it. If the queue is empty, `pop_front` will return `None`.
You can also check the length of the queue using the `len` method:
let queue_length = queue.len();
This returns the number of elements currently in the queue.
Here is an example program that demonstrates how to use a queue in Rust:
use std::collections::VecDeque;
fn main() {
let mut queue = VecDeque::new();
queue.push_back(1);
queue.push_back(2);
queue.push_back(3);
while let Some(element) = queue.pop_front() {
println!("Element: {}", element);
}
}
This program creates a new queue, adds three elements to it, and then removes and prints each element in turn. The output of this program will be:
Element: 1
Element: 2
Element: 3
What is a Queue in Rust?
In conclusion, a queue is a fundamental data structure that is used to store and manage a collection of elements in a specific order. In Rust, queues can be implemented using various data structures such as arrays, linked lists, and vectors. Rust’s standard library provides a Queue trait that defines the basic operations that a queue should support. These operations include enqueue, dequeue, peek, and is_empty. Queues are commonly used in computer science and software engineering to solve a wide range of problems, such as scheduling tasks, managing resources, and implementing algorithms. By understanding the concept of queues in Rust, developers can build efficient and reliable software applications that can handle complex data processing tasks.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |