A queue is a linear data structure in computer science that follows the First-In-First-Out (FIFO) principle. It is similar to a line of people waiting for a service, where the first person to join the line is the first to be served. In a queue, elements are added at the rear end and removed from the front end. The operations performed on a queue are enqueue, which adds an element to the rear end, and dequeue, which removes an element from the front end. Queues are commonly used in computer algorithms, such as breadth-first search and job scheduling, where the order of processing is important. Keep reading below to learn how to use a Queue in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
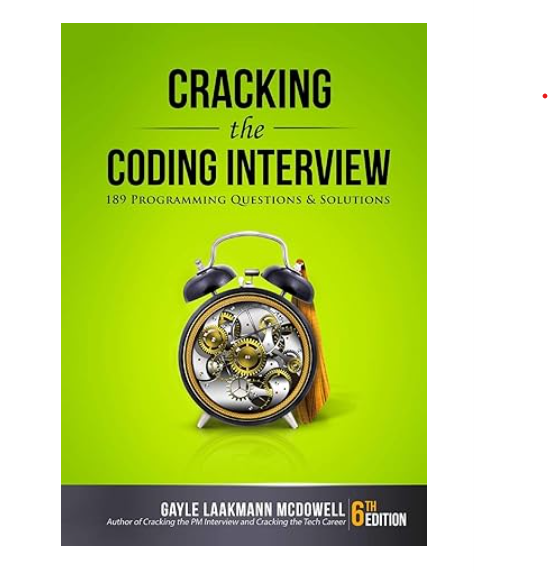
How to use a Queue in TypeScript with example code
Queues are a fundamental data structure in computer science that allow us to store and manage a collection of elements in a specific order. In TypeScript, we can implement a Queue using an array and a few simple methods. In this blog post, we will explore how to use a Queue in TypeScript with example code.
To begin, let’s define what a Queue is. A Queue is a data structure that follows the First-In-First-Out (FIFO) principle. This means that the first element added to the Queue will be the first element removed from the Queue. Think of a Queue as a line of people waiting for a service. The first person in line will be the first person to receive the service, and so on.
In TypeScript, we can implement a Queue using an array and two simple methods: `enqueue` and `dequeue`. The `enqueue` method adds an element to the end of the Queue, while the `dequeue` method removes the first element from the Queue.
Here is an example implementation of a Queue in TypeScript:
class Queue {
private elements: any[] = [];
enqueue(element: any) {
this.elements.push(element);
}
dequeue() {
return this.elements.shift();
}
}
In this implementation, we define a `Queue` class with a private `elements` array. The `enqueue` method adds an element to the end of the `elements` array using the `push` method. The `dequeue` method removes the first element from the `elements` array using the `shift` method.
Let’s see how we can use this Queue in TypeScript. Suppose we want to implement a program that simulates a line of people waiting for a rollercoaster ride. We can use a Queue to manage the line of people. Here is an example implementation:
const rollercoasterQueue = new Queue();
rollercoasterQueue.enqueue("Alice");
rollercoasterQueue.enqueue("Bob");
rollercoasterQueue.enqueue("Charlie");
console.log(rollercoasterQueue.dequeue()); // Output: Alice
console.log(rollercoasterQueue.dequeue()); // Output: Bob
console.log(rollercoasterQueue.dequeue()); // Output: Charlie
In this example, we create a new `Queue` called `rollercoasterQueue`. We then add three people to the Queue using the `enqueue` method. Finally, we remove each person from the Queue using the `dequeue` method and print their names to the console.
In conclusion, Queues are a powerful data structure that can be used to manage collections of elements in a specific order. In TypeScript, we can implement a Queue using an array and two simple methods: `enqueue` and `dequeue`. By using a Queue, we can easily manage lines of people, process tasks in a specific order, and much more.
What is a Queue in TypeScript?
In conclusion, a Queue in TypeScript is a data structure that follows the First-In-First-Out (FIFO) principle. It is a collection of elements that can be added to the back and removed from the front. Queues are commonly used in computer science and programming to manage tasks, events, and processes. TypeScript provides a built-in Queue class that can be used to implement this data structure in your code. By understanding the concept of Queues and how to use them in TypeScript, you can improve the efficiency and organization of your programs. Whether you are a beginner or an experienced developer, Queues are an essential tool to have in your programming arsenal.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |