In computer science, a Set is a data structure that stores a collection of unique elements. It is typically implemented using a hash table or a binary search tree, and provides efficient operations for adding, removing, and checking for the presence of elements. Sets are commonly used in algorithms and data processing tasks where duplicate elements are not allowed or need to be removed. They are also used in database systems to enforce uniqueness constraints on data. Keep reading below to learn how to use a Set in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
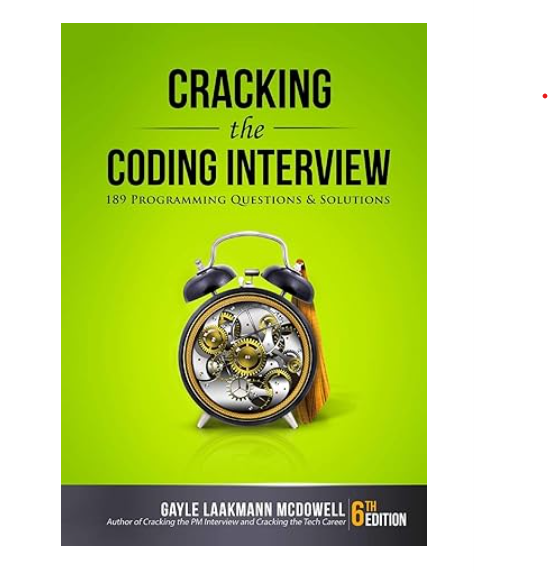
How to use a Set in Go with example code
A Set is a collection of unique elements in Go. It is similar to a slice, but it does not allow duplicates. In this blog post, we will explore how to use a Set in Go with example code.
To use a Set in Go, we need to import the “container/set” package. This package provides a Set data structure that we can use in our code. Here is an example of how to create a Set:
import "container/set"
func main() {
s := set.New()
}
In this example, we create a new Set using the “set.New()” function. This function returns a new empty Set that we can use to store our elements.
To add elements to the Set, we can use the “s.Insert()” function. Here is an example:
s.Insert(1)
s.Insert(2)
s.Insert(3)
In this example, we add three elements to the Set: 1, 2, and 3. Since Sets do not allow duplicates, if we try to add an element that already exists in the Set, it will be ignored.
To remove elements from the Set, we can use the “s.Remove()” function. Here is an example:
s.Remove(2)
In this example, we remove the element 2 from the Set.
To check if an element exists in the Set, we can use the “s.Has()” function. Here is an example:
if s.Has(1) {
fmt.Println("Set contains 1")
}
In this example, we check if the Set contains the element 1. If it does, we print a message to the console.
Finally, to get the number of elements in the Set, we can use the “s.Len()” function. Here is an example:
fmt.Println("Set length:", s.Len())
In this example, we print the length of the Set to the console.
In conclusion, Sets are a useful data structure in Go for storing unique elements. By using the “container/set” package, we can easily create and manipulate Sets in our code.
What is a Set in Go?
In conclusion, a set in Go is a collection of unique elements that are unordered and cannot be duplicated. It is a useful data structure that can be used to efficiently store and manipulate data in various applications. Go provides a built-in map type that can be used to implement a set, and there are also third-party packages available that provide additional functionality for working with sets. Whether you are a beginner or an experienced Go developer, understanding the concept of sets and how to use them can greatly enhance your programming skills and help you build more efficient and effective applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |